用c++的方法设计递归算法判断字符串是否为回文。 采用字符型数组存放字符串,设计一个递归算法,判断字符串 str 是否为回 文。
时间: 2023-10-26 20:06:48 浏览: 176
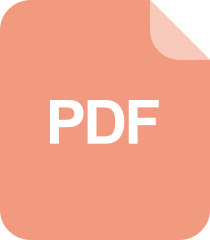
后缀数组(处理字符串的有力工具)
以下是递归算法的实现,基本思路是判断字符串的第一个字符和最后一个字符是否相等,如果相等则递归判断去掉首尾字符的字符串是否为回文。
```c++
#include <iostream>
#include <cstring>
using namespace std;
bool isPalindrome(char str[], int start, int end) {
if(start >= end) { // 递归边界:字符串长度为0或1时,一定是回文
return true;
}
if(str[start] != str[end]) { // 如果首尾字符不相等,则一定不是回文
return false;
}
return isPalindrome(str, start+1, end-1); // 递归判断去掉首尾字符后的字符串是否为回文
}
int main() {
char str[100];
cin >> str;
int len = strlen(str);
if(isPalindrome(str, 0, len-1)) {
cout << "Yes" << endl;
} else {
cout << "No" << endl;
}
return 0;
}
```
注意,在调用递归函数时,传入的start和end参数分别代表字符串的起始位置和结束位置,初始时应为0和len-1,其中len为字符串的长度。
阅读全文
相关推荐
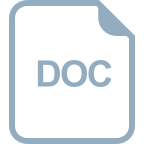
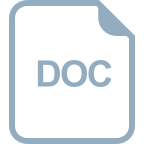


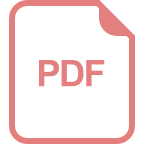
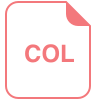

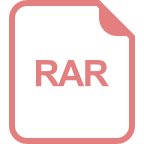
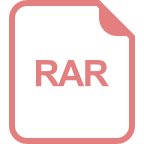
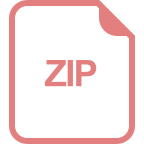
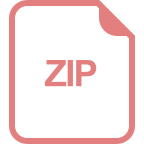
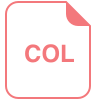
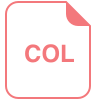
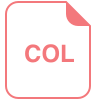
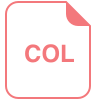


