Invalid hook call. Hooks can only be called inside of the body of a function component. This could happen for one of the following reasons:
时间: 2024-05-12 22:15:04 浏览: 193
This error message usually occurs when you try to use a React hook outside of a function component or inside a conditional statement or loop. React hooks are meant to be used only inside function components and not inside class components or plain JavaScript functions.
To fix this error, make sure that you are using the hook inside a function component and that the component is properly defined. Also, ensure that you are not calling the hook conditionally or in a loop.
Here's an example of how to use a hook correctly inside a function component:
```
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
function handleClick() {
setCount(count + 1);
}
return (
<div>
<p>You clicked {count} times</p>
<button onClick={handleClick}>Click me</button>
</div>
);
}
export default MyComponent;
```
In this example, the `useState` hook is used inside the `MyComponent` function to define a state variable `count` and its corresponding update function `setCount`. The `handleClick` function uses the `setCount` function to update the `count` state when the button is clicked. Finally, the component returns some JSX that displays the current count and a button that triggers the `handleClick` function.
阅读全文
相关推荐
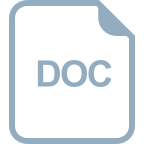
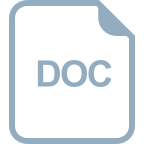
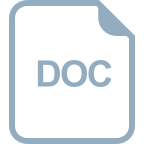


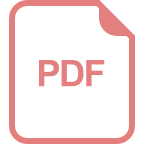
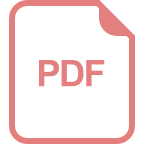
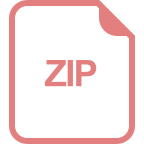
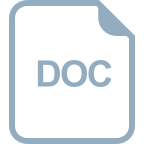
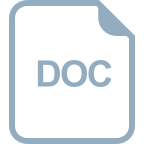
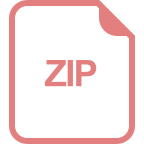