设计一个圆类 circle, 一个方类 square 和一个桌子类 table,另设 计一个圆桌类 roundtable 和一个方桌类 squaretable,分别是从两 个类派生得到的,要求输出两类桌子的高度、面积、长宽(直径)、 颜色、重量、材质和价格等数据。
时间: 2024-02-05 16:02:43 浏览: 118
好的,我可以帮您设计这些类和实现这些要求。以下是一个可能的实现:
```python
class Circle:
def __init__(self, radius, color, weight, material, price):
self.radius = radius
self.color = color
self.weight = weight
self.material = material
self.price = price
def area(self):
return 3.14 * self.radius ** 2
def diameter(self):
return 2 * self.radius
class Square:
def __init__(self, side, color, weight, material, price):
self.side = side
self.color = color
self.weight = weight
self.material = material
self.price = price
def area(self):
return self.side ** 2
def length(self):
return self.side
def width(self):
return self.side
class Table:
def __init__(self, height, color, weight, material, price):
self.height = height
self.color = color
self.weight = weight
self.material = material
self.price = price
class RoundTable(Table, Circle):
def __init__(self, radius, height, color, weight, material, price):
Table.__init__(self, height, color, weight, material, price)
Circle.__init__(self, radius, color, weight, material, price)
class SquareTable(Table, Square):
def __init__(self, side, height, color, weight, material, price):
Table.__init__(self, height, color, weight, material, price)
Square.__init__(self, side, color, weight, material, price)
```
这里定义了一个圆类 `Circle`,一个方类 `Square` 和一个桌子类 `Table`。`Circle` 和 `Square` 类都有 `area` 方法和一些其他方法来计算直径、颜色、重量、材质和价格等属性。`Table` 类只有高度、颜色、重量、材质和价格等属性。
然后,我们定义了两个子类:`RoundTable` 和 `SquareTable`。这些类都是从两个类派生得到的。`RoundTable` 类从 `Table` 和 `Circle` 类派生而来,而 `SquareTable` 类从 `Table` 和 `Square` 类派生而来。这些子类都有自己的构造函数,可以调用父类的构造函数,并在必要时添加自己的属性。
以下是一个使用这些类的例子:
```python
c = Circle(radius=5, color='red', weight=10, material='plastic', price=20)
print('Circle diameter:', c.diameter())
print('Circle color:', c.color)
print('Circle area:', c.area())
s = Square(side=4, color='blue', weight=8, material='wood', price=30)
print('Square length:', s.length())
print('Square width:', s.width())
print('Square color:', s.color)
print('Square area:', s.area())
rt = RoundTable(radius=6, height=2, color='green', weight=15, material='metal', price=50)
print('Round table diameter:', rt.diameter())
print('Round table color:', rt.color)
print('Round table height:', rt.height)
print('Round table area:', rt.area())
st = SquareTable(side=3, height=2, color='yellow', weight=12, material='glass', price=40)
print('Square table length:', st.length())
print('Square table width:', st.width())
print('Square table color:', st.color)
print('Square table height:', st.height)
print('Square table area:', st.area())
```
这个例子创建了一个圆对象 `c`,一个方对象 `s`,一个圆桌对象 `rt` 和一个方桌对象 `st`。它们分别输出了直径、颜色、高度、面积、长度、宽度、重量、材质和价格等属性。
阅读全文
相关推荐
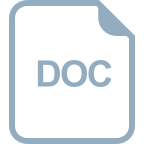
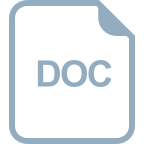
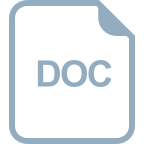


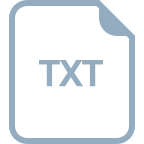
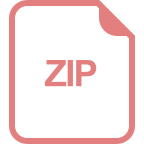
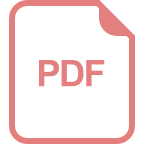
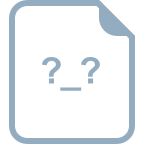
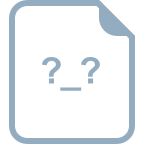
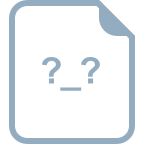
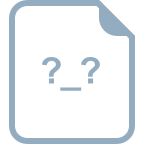
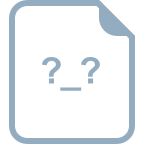
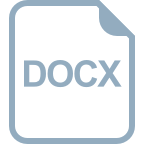
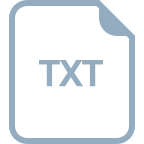
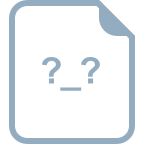
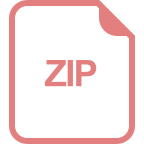
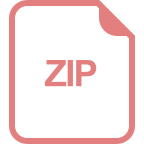