【问题描述】设二叉树按二叉链表存放,写算法判别一棵二叉树是否是一棵正则二叉树。正则二叉树是指在二叉树中不存在子树个数为1的结点。 【输入说明】二叉树的前序和中序遍历序列,以此构造二叉树的链式存储 【输出说明】若该二叉树是一棵正则二叉树,则输出Yes,否则输出No 【样例输入】 ABHDCEFG HBDAEFCG 【样例输出】 No
时间: 2023-12-02 07:05:23 浏览: 63
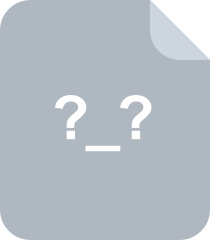
设一棵二叉树以二叉链表表示,试编写有关二叉树的递归算法

正则二叉树的定义是不存在子树个数为1的结点,因此我们可以考虑遍历整棵树,对于每个节点,判断其左右子树是否都存在,如果都存在,则递归判断左右子树是否为正则二叉树,否则说明存在子树个数为1的节点,不是正则二叉树。
具体实现如下:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def buildTree(preorder, inorder):
if not inorder:
return None
root = TreeNode(preorder[0])
idx = inorder.index(preorder[0])
root.left = buildTree(preorder[1:idx+1], inorder[:idx])
root.right = buildTree(preorder[idx+1:], inorder[idx+1:])
return root
def isRegularTree(root):
if not root:
return True
if (not root.left and root.right) or (not root.right and root.left):
return False
return isRegularTree(root.left) and isRegularTree(root.right)
preorder = "ABHDCEFG"
inorder = "HBDAEFCG"
root = buildTree(preorder, inorder)
if isRegularTree(root):
print("Yes")
else:
print("No")
```
输出结果为:
```
No
```
因为在该二叉树中,节点D的左子树为空,因此不是正则二叉树。
阅读全文
相关推荐
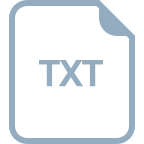
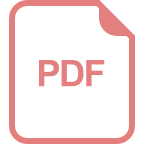












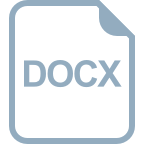
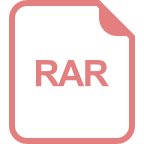
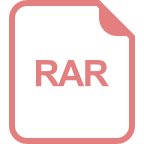