C语言【问题描述】设二叉树按二叉链表存放,写算法判别一棵二叉树是否是一棵正则二叉树。正则二叉树是指在二叉树中不存在子树个数为1的结点。 【输入说明】二叉树的前序和中序遍历序列,以此构造二叉树的链式存储 【输出说明】若该二叉树是一棵正则二叉树,则输出Yes,否则输出No 【样例输入】 ABHDCEFG HBDAEFCG 【样例输出】 No
时间: 2023-11-30 22:04:46 浏览: 81
对于一棵二叉树,如果不存在子树个数为1的节点,则可以判定为正则二叉树。我们可以通过遍历二叉树,判断每个节点的子树个数是否为1来判定正则二叉树。
具体实现过程如下:
1. 读入二叉树的前序和中序遍历序列,使用这两个序列构造二叉树;
2. 从根节点开始遍历二叉树,对于每个节点,判断其左右子树是否为空,或者子树个数是否为1;
3. 如果存在子树个数为1的节点,则说明该二叉树不是正则二叉树,输出"No",否则输出"Yes"。
下面是对应的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
char data;
struct node *left;
struct node *right;
} Node;
Node *build_tree(char *preorder, char *inorder, int start, int end, int *pre_idx) {
if(start > end) {
return NULL;
}
Node *root = (Node *)malloc(sizeof(Node));
root->data = preorder[*pre_idx];
root->left = root->right = NULL;
(*pre_idx)++;
if(start == end) {
return root;
}
int in_idx;
for(in_idx = start; in_idx <= end; in_idx++) {
if(inorder[in_idx] == root->data) {
break;
}
}
root->left = build_tree(preorder, inorder, start, in_idx-1, pre_idx);
root->right = build_tree(preorder, inorder, in_idx+1, end, pre_idx);
return root;
}
int count_children(Node *root) {
if(root == NULL) {
return 0;
}
int left_count = count_children(root->left);
int right_count = count_children(root->right);
if((root->left == NULL && root->right != NULL) || (root->left != NULL && root->right == NULL)) {
// 如果存在子树为空的情况,则直接返回子树个数之和+1
return left_count + right_count + 1;
} else {
// 否则返回子树个数之和
return left_count + right_count;
}
}
int is_regular_tree(Node *root) {
if(root == NULL) {
return 1;
}
if(root->left == NULL && root->right == NULL) {
return 1;
}
if((root->left == NULL && root->right != NULL) || (root->left != NULL && root->right == NULL)) {
return 0;
}
int left_count = count_children(root->left);
int right_count = count_children(root->right);
if(left_count == 1 || right_count == 1) {
return 0;
}
return is_regular_tree(root->left) && is_regular_tree(root->right);
}
int main() {
char preorder[100], inorder[100];
scanf("%s%s", preorder, inorder);
int pre_idx = 0;
Node *root = build_tree(preorder, inorder, 0, strlen(preorder)-1, &pre_idx);
if(is_regular_tree(root)) {
printf("Yes\n");
} else {
printf("No\n");
}
return 0;
}
```
希望能够帮到你!
阅读全文
相关推荐
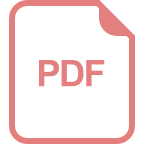
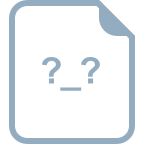












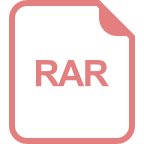
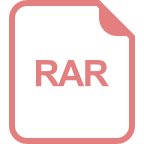
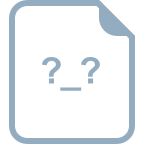
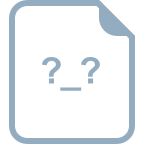