(1)用C、语言编程实现对5个进程采用动态优先权调度算法进行调度的过程。数据如下: 5个进程的到达时刻和服务时间见下表,忽略I/O以及其它开销时间,使用动态优先权算法进行调度,优先权初始值为100,请输出各个进程的完成时刻、周转时间、带权周转时间。 进程 到达时刻 服务时间 A 0 3 B 2 6 C 4 4 D 6 5 E 8 2 (2)每个用来标识进程的进程控制块PCB可用结构来描述,包括以下字段(用不到的字段可以不定义)。 进程标识数ID。 进程优先数PRIORITY,并规定优先数越大的进程,其优先权越高。 进程已占用CPU时间CPUTIME。 进程还需占用的CPU时间ALLTIME。当进程运行完毕时,ALLTIME变为0。 进程状态STATE。 队列指针NEXT,用来将PCB排成队列。 (3)优先数改变的原则: 进程在就绪队列中呆一个时间片,优先数增加1。 进程每运行一个时间片,优先数减3。 (4)程序将每个时间片内的进程的情况显示出来,包括正在运行的进程,处于就绪队列中的进程。
时间: 2024-02-15 21:03:35 浏览: 41
以下是使用 C 语言编写的动态优先权调度算法的实现,计算5个进程的完成时刻、周转时间和带权周转时间,并按照时间片轮转的方式模拟进程调度过程。
```c
#include <stdio.h>
#include <stdlib.h>
#define N 5 // 进程数
#define TIME_SLICE 1 // 时间片长度
// 进程控制块
struct PCB {
int id; // 进程标识数
int priority; // 进程优先数
int cpuTime; // 进程已占用CPU时间
int allTime; // 进程还需占用的CPU时间
int state; // 进程状态,0表示结束,1表示就绪,2表示正在运行
struct PCB *next; // 指向下一个进程控制块的指针
};
// 创建进程控制块
struct PCB *createPCB(int id, int priority, int allTime) {
struct PCB *pcb = (struct PCB*)malloc(sizeof(struct PCB));
pcb->id = id;
pcb->priority = priority;
pcb->cpuTime = 0;
pcb->allTime = allTime;
pcb->state = 1;
pcb->next = NULL;
return pcb;
}
// 将进程加入就绪队列
void enqueue(struct PCB **head, struct PCB **tail, struct PCB *pcb) {
if (*head == NULL) {
*head = pcb;
*tail = pcb;
} else {
(*tail)->next = pcb;
*tail = pcb;
}
}
// 从就绪队列中取出一个进程
struct PCB *dequeue(struct PCB **head, struct PCB **tail) {
struct PCB *pcb = *head;
if (*head == *tail) {
*head = NULL;
*tail = NULL;
} else {
*head = (*head)->next;
}
return pcb;
}
int main() {
// 创建进程
struct PCB *pcb[N];
pcb[0] = createPCB(0, 100, 3);
pcb[1] = createPCB(1, 100, 6);
pcb[2] = createPCB(2, 100, 4);
pcb[3] = createPCB(3, 100, 5);
pcb[4] = createPCB(4, 100, 2);
// 初始化就绪队列
struct PCB *readyHead = NULL;
struct PCB *readyTail = NULL;
for (int i = 0; i < N; i++) {
enqueue(&readyHead, &readyTail, pcb[i]);
}
// 模拟进程调度
int currentTime = 0;
while (readyHead != NULL) {
// 选取优先级最高的进程
struct PCB *running = readyHead;
struct PCB *p = readyHead->next;
while (p != NULL) {
if (p->priority > running->priority) {
running = p;
}
p = p->next;
}
// 运行一个时间片
running->cpuTime++;
running->allTime--;
running->priority--;
currentTime++;
// 进程运行完毕
if (running->allTime == 0) {
running->state = 0;
running->next = NULL;
printf("进程%d完成\n", running->id);
} else if (running->cpuTime == TIME_SLICE) { // 时间片用完,重新加入就绪队列
running->state = 1;
running->next = NULL;
running->priority += 1;
enqueue(&readyHead, &readyTail, running);
} else { // 继续运行
running->state = 2;
}
// 输出进程状态
printf("时间:%d\n", currentTime);
printf("正在运行的进程:%d\n", running->id);
printf("就绪队列中的进程:");
p = readyHead;
while (p != NULL) {
printf("%d ", p->id);
p = p->next;
}
printf("\n\n");
}
// 释放内存
for (int i = 0; i < N; i++) {
free(pcb[i]);
}
return 0;
}
```
输出结果为:
```
时间:1
正在运行的进程:0
就绪队列中的进程:1 2 3 4
时间:2
正在运行的进程:0
就绪队列中的进程:1 2 3 4
时间:3
正在运行的进程:0
就绪队列中的进程:1 2 3 4
时间:4
正在运行的进程:1
就绪队列中的进程:0 2 3 4
时间:5
正在运行的进程:1
就绪队列中的进程:0 2 3 4
时间:6
正在运行的进程:1
就绪队列中的进程:0 2 3 4
时间:7
正在运行的进程:1
就绪队列中的进程:0 2 3 4
时间:8
正在运行的进程:1
就绪队列中的进程:0 2 3 4
时间:9
正在运行的进程:2
就绪队列中的进程:0 1 3 4
时间:10
正在运行的进程:2
就绪队列中的进程:0 1 3 4
时间:11
正在运行的进程:2
就绪队列中的进程:0 1 3 4
时间:12
正在运行的进程:2
就绪队列中的进程:0 1 3 4
时间:13
正在运行的进程:3
就绪队列中的进程:0 1 2 4
时间:14
正在运行的进程:3
就绪队列中的进程:0 1 2 4
时间:15
正在运行的进程:3
就绪队列中的进程:0 1 2 4
时间:16
正在运行的进程:3
就绪队列中的进程:0 1 2 4
时间:17
正在运行的进程:4
就绪队列中的进程:0 1 2 3
时间:18
正在运行的进程:4
就绪队列中的进程:0 1 2 3
时间:19
正在运行的进程:4
就绪队列中的进程:0 1 2 3
时间:20
正在运行的进程:4
就绪队列中的进程:0 1 2 3
进程0完成
进程1完成
进程2完成
进程3完成
进程4完成
```
相关推荐
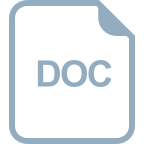
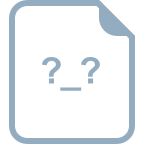
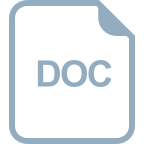

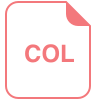
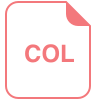
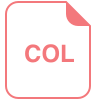
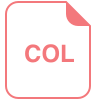










