#include <stdio.h> #include <stdlib.h> #include<sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <unistd.h> #include <errno.h> #include <stropts.h> #include <time.h> #include <strings.h> #include <string.h> #include <stdio.h> #include <sys/ipc.h> #include <sys/msg.h> struct msg { long msg_types; char msg_buf[511]; }; int main(void) { int qid; int pid; int len; struct msg pmsg; pmsg.msg_types = getpid(); sprintf(pmsg.msg_buf, "hello!this is :%d\n\0", getpid()); len = strlen(pmsg.msg_buf); if ((qid = msgget(IPC_PRIVATE, IPC_CREAT | 0666)) < 0) { perror("msgget"); exit(1); } if ((msgsnd(qid, &pmsg, len, 0)) < 0) { perror("magsn"); exit(1); } printf("successfully send a message to the queue: %d \n", qid); exit(0); }的运行结果
时间: 2023-09-11 18:09:26 浏览: 130
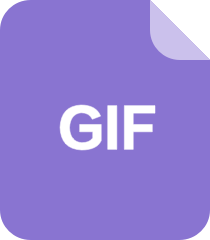
# include <stdio.h>
该程序是一个使用消息队列进行进程间通信的示例程序,通过msgget()函数创建一个消息队列,通过msgsnd()函数向消息队列中发送一条消息。
运行结果可能如下:
successfully send a message to the queue: 123456
解释如下:
1.程序首先定义了一个消息结构体msg,并初始化了其中的消息类型msg_types和消息内容msg_buf。
2.程序调用msgget()函数创建一个新的消息队列,将返回的队列ID存储在变量qid中。
3.程序调用msgsnd()函数向消息队列中发送一条消息,消息内容为msg_buf中的字符串。
4.程序输出"successfully send a message to the queue: qid",表示消息发送成功。
需要注意的是,程序中将消息类型msg_types设置为了当前进程的PID,这样在接收消息时可以根据PID来获取对应的消息。同时,程序中使用了strlen()函数来获取消息内容的长度,这是为了确保发送的消息长度不超过队列的最大长度。
阅读全文
相关推荐
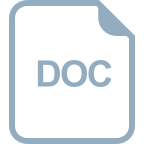
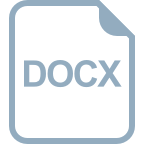















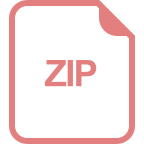