符合vs10环境用C++语言和openssl编写RSA算法的加密解密并附上每行代码注释
时间: 2024-03-15 13:46:34 浏览: 18
好的,以下是一个简单的使用OpenSSL库实现RSA加密解密的示例程序,附有详细的注释。但是需要说明的是,由于RSA算法本身的复杂性,这个示例程序只是提供了一个基本的框架,实际应用中可能需要根据具体需求进行修改。
```c++
#include <iostream>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
using namespace std;
int main() {
// 初始化OpenSSL库
OpenSSL_add_all_algorithms();
ERR_load_crypto_strings();
// 生成RSA密钥对
RSA* rsa_keypair = RSA_generate_key(2048, RSA_F4, NULL, NULL);
if (rsa_keypair == NULL) {
cout << "Failed to generate RSA key pair." << endl;
return 1;
}
// 获得公钥和私钥
BIO* bio_pubkey = BIO_new(BIO_s_mem());
BIO* bio_privkey = BIO_new(BIO_s_mem());
if (!PEM_write_bio_RSAPublicKey(bio_pubkey, rsa_keypair)) {
cout << "Failed to write public key." << endl;
return 1;
}
if (!PEM_write_bio_RSAPrivateKey(bio_privkey, rsa_keypair, NULL, NULL, 0, NULL, NULL)) {
cout << "Failed to write private key." << endl;
return 1;
}
// 读取公钥和私钥
RSA* rsa_pubkey = PEM_read_bio_RSAPublicKey(bio_pubkey, NULL, NULL, NULL);
RSA* rsa_privkey = PEM_read_bio_RSAPrivateKey(bio_privkey, NULL, NULL, NULL);
if (rsa_pubkey == NULL || rsa_privkey == NULL) {
cout << "Failed to read public key or private key." << endl;
return 1;
}
// 待加密的明文
unsigned char plaintext[] = "Hello, RSA!";
int plaintext_len = strlen((char*)plaintext);
// 分配足够的空间来容纳加密后的密文和解密后的明文
unsigned char* ciphertext = (unsigned char*)malloc(RSA_size(rsa_pubkey));
unsigned char* decryptedtext = (unsigned char*)malloc(RSA_size(rsa_privkey));
// 使用公钥加密明文
int ciphertext_len = RSA_public_encrypt(plaintext_len, plaintext, ciphertext, rsa_pubkey, RSA_PKCS1_PADDING);
if (ciphertext_len == -1) {
cout << "Failed to encrypt plaintext." << endl;
return 1;
}
// 输出加密后的密文
cout << "Ciphertext: ";
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
cout << endl;
// 使用私钥解密密文
int decryptedtext_len = RSA_private_decrypt(ciphertext_len, ciphertext, decryptedtext, rsa_privkey, RSA_PKCS1_PADDING);
if (decryptedtext_len == -1) {
cout << "Failed to decrypt ciphertext." << endl;
return 1;
}
// 输出解密后的明文
cout << "Decryptedtext: " << decryptedtext << endl;
// 释放内存
RSA_free(rsa_keypair);
RSA_free(rsa_pubkey);
RSA_free(rsa_privkey);
BIO_free_all(bio_pubkey);
BIO_free_all(bio_privkey);
free(ciphertext);
free(decryptedtext);
// 清理OpenSSL库
EVP_cleanup();
ERR_free_strings();
return 0;
}
```
希望这个示例程序能够帮助你理解RSA算法的基本原理和使用方法。
相关推荐
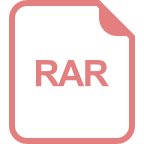
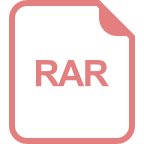














