rsa加密解密算法c++语言
时间: 2023-08-13 07:25:11 浏览: 80
RSA是一种非对称加密算法,下面给出RSA加密解密算法的C++代码实现。
1. 生成RSA密钥对
```cpp
#include <openssl/rsa.h>
#include <openssl/pem.h>
void generateRSAKeyPair(RSA *&publicKey, RSA *&privateKey) {
// 随机数种子
srand((unsigned)time(NULL));
// 生成RSA密钥对
int bits = 1024; // 密钥长度
unsigned long e = RSA_F4;
publicKey = RSA_new();
privateKey = RSA_new();
BIGNUM *bn = BN_new();
BN_set_word(bn, e);
RSA_generate_key_ex(publicKey, bits, bn, NULL);
RSA_generate_key_ex(privateKey, bits, bn, NULL);
BN_free(bn);
}
```
2. RSA公钥加密
```cpp
#include <openssl/rsa.h>
#include <openssl/pem.h>
std::string rsaPublicKeyEncrypt(RSA *publicKey, const std::string &plainText) {
int rsaSize = RSA_size(publicKey);
int blockSize = rsaSize - 11;
int blockNum = (plainText.length() + blockSize - 1) / blockSize;
std::string cipherText;
for (int i = 0; i < blockNum; i++) {
std::string plainBlock = plainText.substr(i * blockSize, blockSize);
char *cipherBlock = new char[rsaSize];
int len = RSA_public_encrypt(plainBlock.length(), (const unsigned char*)plainBlock.c_str(), (unsigned char*)cipherBlock, publicKey, RSA_PKCS1_PADDING);
cipherText.append(cipherBlock, len);
delete[] cipherBlock;
}
return cipherText;
}
```
3. RSA私钥解密
```cpp
#include <openssl/rsa.h>
#include <openssl/pem.h>
std::string rsaPrivateKeyDecrypt(RSA *privateKey, const std::string &cipherText) {
int rsaSize = RSA_size(privateKey);
int blockSize = rsaSize;
int blockNum = (cipherText.length() + blockSize - 1) / blockSize;
std::string plainText;
for (int i = 0; i < blockNum; i++) {
std::string cipherBlock = cipherText.substr(i * blockSize, blockSize);
char *plainBlock = new char[rsaSize];
int len = RSA_private_decrypt(cipherBlock.length(), (const unsigned char*)cipherBlock.c_str(), (unsigned char*)plainBlock, privateKey, RSA_PKCS1_PADDING);
plainText.append(plainBlock, len);
delete[] plainBlock;
}
return plainText;
}
```
使用时,可以先生成RSA密钥对,然后使用公钥加密数据,再使用私钥解密数据。具体代码如下:
```cpp
// 生成RSA密钥对
RSA *publicKey = NULL, *privateKey = NULL;
generateRSAKeyPair(publicKey, privateKey);
// 使用公钥加密数据
std::string plainText = "Hello, RSA!";
std::string cipherText = rsaPublicKeyEncrypt(publicKey, plainText);
// 使用私钥解密数据
std::string decryptedText = rsaPrivateKeyDecrypt(privateKey, cipherText);
std::cout << decryptedText << std::endl;
// 释放RSA密钥对
RSA_free(publicKey);
RSA_free(privateKey);
```
相关推荐
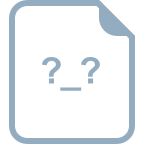
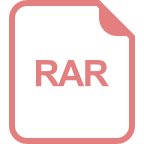














