这段代码有什么问题:#include<bits/stdc++.h> using namespace std; int main() { string S, s1,str; char s2, x, y; int n = 0, index, start, end, find_index; size_t pos; while (cin >> S) { cin >> s1 >> index >> s2 >> x >> y >> start >> end >> str; n++; cout << "Case " << n << ":" << endl; //insert S.insert(index, s1); cout << "Insert->" << S << endl; //erase for (int i = 0; i < S.length(); i++) { if (S[i] == s2) S.erase(i, 1); } cout << "Erase->" << S << endl; //replace for (int i = 0; i < S.length(); i++) { if (S[i] == x) S.replace(i,i, 1, y); } cout << "Replace->" << S << endl; //size cout << "Size->" << S.size() << endl; //reverse string temp = S; reverse(temp.begin(), temp.end()); cout << "Reverse->" << temp << endl; //sub cout << "Sub->" << S.substr(start, end - start + 1) << endl; //find find_index = S.find(str); if (find_index != string::npos) cout << "Find->" << find_index << endl; else cout << "Find->" << -1 << endl; } return 0; }
时间: 2023-12-11 07:03:20 浏览: 141
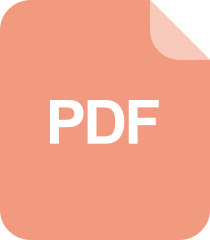
万能头文件#include“bitsstdc++.h”.pdf
这段代码存在以下问题:
1. `#include<bits/stdc++.h>` 这条语句不是标准的 C++ 头文件引用语句,会引入很多不必要的头文件,应该避免使用。
2. `using namespace std;` 这条语句会将 std 命名空间中的所有函数、变量等引入到当前作用域中,容易引起命名冲突和命名空间污染,应该避免使用。
3. 在使用 `S.replace(i, i, 1, y)` 进行替换时,第二个参数应该是被替换子串的长度,而不是起始位置,这里应该改为 `S.replace(i, 1, 1, y)`。
4. 在使用 `S.find(str)` 进行查找时,如果没有找到匹配的子串,`find()` 函数会返回 `string::npos`,应该使用 `!=` 进行判断,而不是 `==`。
5. 在使用 `S.substr(start, end - start + 1)` 进行子串截取时,应该再加上一个判断,防止截取范围超出字符串的实际长度。
建议改进的代码如下:
```
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string S, s1, str;
char s2, x, y;
int n = 0, index, start, end, find_index;
while (std::cin >> S) {
std::cin >> s1 >> index >> s2 >> x >> y >> start >> end >> str;
n++;
std::cout << "Case " << n << ":" << std::endl;
// insert
S.insert(index, s1);
std::cout << "Insert->" << S << std::endl;
// erase
S.erase(std::remove(S.begin(), S.end(), s2), S.end());
std::cout << "Erase->" << S << std::endl;
// replace
for (int i = 0; i < S.length(); i++) {
if (S[i] == x) {
S.replace(i, 1, 1, y);
}
}
std::cout << "Replace->" << S << std::endl;
// size
std::cout << "Size->" << S.size() << std::endl;
// reverse
std::string temp = S;
std::reverse(temp.begin(), temp.end());
std::cout << "Reverse->" << temp << std::endl;
// sub
if (start >= 0 && start < S.length() && end >= 0 && end < S.length() && end >= start) {
std::cout << "Sub->" << S.substr(start, end - start + 1) << std::endl;
} else {
std::cout << "Sub->" << "invalid range" << std::endl;
}
// find
find_index = S.find(str);
if (find_index != std::string::npos) {
std::cout << "Find->" << find_index << std::endl;
} else {
std::cout << "Find->" << -1 << std::endl;
}
}
return 0;
}
```
阅读全文
相关推荐
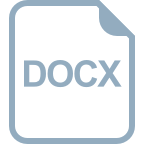
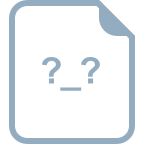
















