android开发kotlin使用layoutinflater获取view
时间: 2024-03-23 07:38:25 浏览: 17
在 Kotlin 中使用 LayoutInflater 获取 View 的方法与 Java 中基本相同。可以使用以下代码来获取 View:
```kotlin
val layoutInflater = LayoutInflater.from(context)
val view = layoutInflater.inflate(R.layout.my_layout, parent, false)
```
其中,`context` 是当前的上下文,`my_layout` 是要加载的布局文件的名称,`parent` 是可选的 ViewGroup,用于指定要将布局文件添加到哪个父布局中。`false` 表示不将新创建的 View 添加到父 ViewGroup 中,因为我们会在其他地方手动添加它。
请注意,`R.layout.my_layout` 中的 `my_layout` 应该是你的布局文件的名称,需要与你的项目中的实际布局文件名称相匹配。
相关问题
使用kotlin实现android收藏
好的,下面是一个使用Kotlin实现Android收藏的简单示例:
1. 创建一个名为“FavoriteItem”的数据类,用于存储收藏项的信息,比如收藏项的ID、名称、图片等。
```kotlin
data class FavoriteItem(val id: Int, val name: String, val imageUrl: String)
```
2. 创建一个名为“FavoriteAdapter”的RecyclerView适配器,用于显示收藏列表。
```kotlin
class FavoriteAdapter(private val favoriteItems: List<FavoriteItem>) : RecyclerView.Adapter<FavoriteAdapter.ViewHolder>() {
// 创建ViewHolder并绑定布局
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context).inflate(R.layout.favorite_item, parent, false)
return ViewHolder(view)
}
// 获取列表项总数
override fun getItemCount() = favoriteItems.size
// 绑定数据到ViewHolder
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
val favoriteItem = favoriteItems[position]
holder.nameView.text = favoriteItem.name
// 加载图片等操作
}
// ViewHolder类
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val nameView: TextView = itemView.findViewById(R.id.name)
// 其他控件等
}
}
```
3. 在Activity或Fragment中获取收藏列表数据,构建适配器并设置给RecyclerView。
```kotlin
val favoriteItems = listOf(
FavoriteItem(1, "收藏项1", "http://image.url/1.jpg"),
FavoriteItem(2, "收藏项2", "http://image.url/2.jpg"),
FavoriteItem(3, "收藏项3", "http://image.url/3.jpg"),
// 更多收藏项...
)
val recyclerView = findViewById<RecyclerView>(R.id.recyclerView)
recyclerView.layoutManager = LinearLayoutManager(this)
recyclerView.adapter = FavoriteAdapter(favoriteItems)
```
4. 在用户点击收藏按钮时,将收藏项添加到收藏列表中。
```kotlin
// 在收藏按钮的点击事件中调用该方法
fun addToFavorites(id: Int, name: String, imageUrl: String) {
val favoriteItem = FavoriteItem(id, name, imageUrl)
favoriteItems.add(favoriteItem)
favoriteAdapter.notifyDataSetChanged()
}
```
以上是一个简单的收藏功能实现示例,你可以根据实际需求进行修改和扩展。
kotlin-android-extensions 快速迁移到 viewBinding
好的,针对你的问题,我可以给出以下步骤来将已经使用了 `kotlin-android-extensions` 的项目迁移到 `ViewBinding`:
1. 在项目的 build.gradle 中添加以下依赖:
```
android {
...
buildFeatures {
viewBinding true
}
}
dependencies {
...
// 移除 kotlin-android-extensions 依赖
// implementation 'androidx.core:core-ktx:1.3.2'
}
```
2. 在布局文件中添加 `<layout>` 根标签,并使用 ViewBinding 生成的类替代原来的 `findViewById()`。
例如:
```
// 原来的代码
val textView = findViewById<TextView>(R.id.text_view)
// 使用 ViewBinding 生成的类替代
val textView = binding.textView
```
3. 在 Activity 或 Fragment 中使用 ViewBinding 生成的类替代 `setContentView()`。
例如:
```
// 原来的代码
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
...
}
// 使用 ViewBinding 生成的类替代
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
...
}
```
通过以上步骤,你就可以将项目中使用的 `kotlin-android-extensions` 迁移到 `ViewBinding`。希望我的回答能够帮到你。
相关推荐
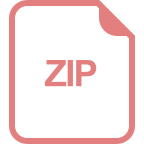
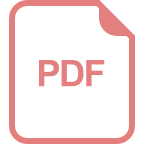
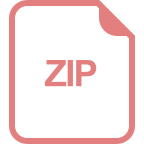












