android kotlin RecyclerView adapter
时间: 2023-11-16 07:06:18 浏览: 98
RecyclerView is a UI component that is used to display large sets of data in an efficient and scrollable manner. The RecyclerView widget is a more advanced and flexible version of ListView. To use RecyclerView, you need to create an adapter that will hold the data and create the views to be displayed. Here is an example of a RecyclerView adapter in Kotlin:
```
class MyAdapter(private val dataList: List<MyData>) : RecyclerView.Adapter<MyAdapter.ViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context).inflate(R.layout.item_layout, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
val data = dataList[position]
holder.bind(data)
}
override fun getItemCount() = dataList.size
inner class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
fun bind(data: MyData) {
// bind data to views
itemView.textViewTitle.text = data.title
itemView.textViewDescription.text = data.description
}
}
}
```
In this example, `MyData` is a data class that holds the data to be displayed in the RecyclerView. The `MyAdapter` class takes a list of `MyData` objects as a parameter in the constructor. The `onCreateViewHolder` method inflates a layout file (`item_layout.xml` in this case) and returns a `ViewHolder` object. The `onBindViewHolder` method binds the data to the views in the `ViewHolder`. Finally, the `getItemCount` method returns the number of items in the list, which is the size of the `dataList`. The `ViewHolder` class holds the views that will be displayed in the RecyclerView and the `bind` method binds the data to the views.
阅读全文
相关推荐
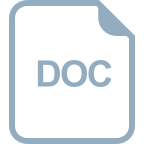
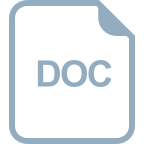
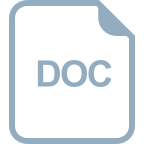




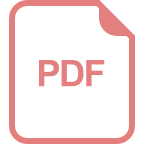

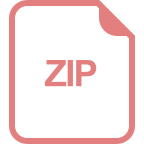








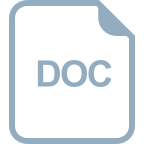