activity kotlin RecyclerView 左滑显示删除按钮 删除item 完整的全部代码实现
时间: 2023-11-29 11:06:33 浏览: 187
好的,以下是一个使用 Kotlin 编写的 RecyclerView 左滑显示删除按钮并删除 item 的完整代码实现:
1. 在你的布局文件中添加 RecyclerView 组件和一个删除按钮的布局(在这里我们使用了 ConstraintLayout):
```
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/delete_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/colorAccent"
android:visibility="gone">
<TextView
android:id="@+id/delete_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="8dp"
android:text="Delete"
android:textColor="@android:color/white"
android:textSize="16sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
2. 创建一个 RecyclerView 的 Adapter,并在 onCreateViewHolder() 方法中设置每个 item 的布局和点击事件。在 onBindViewHolder() 方法中设置每个 item 的数据,并添加滑动删除的逻辑:
```
class MyAdapter(private val list: List<String>) : RecyclerView.Adapter<MyAdapter.ViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context).inflate(R.layout.item_layout, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.itemView.setOnClickListener {
// item 的点击事件
}
holder.bind(list[position])
holder.itemView.setOnTouchListener { v, event ->
when (event.action) {
MotionEvent.ACTION_DOWN -> {
// 记录按下的位置和时间
xDown = event.x
yDown = event.y
timeDown = System.currentTimeMillis()
}
MotionEvent.ACTION_MOVE -> {
// 根据滑动距离和时间计算速度
val xMove = event.x
val yMove = event.y
val distanceX = xMove - xDown
val distanceY = yMove - yDown
val time = System.currentTimeMillis() - timeDown
if (distanceX > touchSlop && Math.abs(distanceY) < touchSlop) {
// 当滑动距离大于 touchSlop 时,显示删除按钮
showDeleteLayout(holder)
return@setOnTouchListener true
} else if (distanceX < -touchSlop && Math.abs(distanceY) < touchSlop) {
// 当滑动距离小于 -touchSlop 时,隐藏删除按钮
hideDeleteLayout(holder)
return@setOnTouchListener true
}
}
MotionEvent.ACTION_UP -> {
// 当手指离开屏幕时,根据滑动距离和时间判断是否删除 item
val xUp = event.x
val yUp = event.y
val distanceX = xUp - xDown
val distanceY = yUp - yDown
val time = System.currentTimeMillis() - timeDown
if (distanceX > touchSlop && Math.abs(distanceY) < touchSlop && time < clickTimeout) {
// 当滑动距离大于 touchSlop,时间小于 clickTimeout,且没有上下滑动时,删除 item
deleteItem(holder)
return@setOnTouchListener true
} else {
// 否则,隐藏删除按钮
hideDeleteLayout(holder)
return@setOnTouchListener true
}
}
}
false
}
}
override fun getItemCount(): Int = list.size
inner class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
fun bind(data: String) {
itemView.findViewById<TextView>(R.id.item_text).text = data
}
}
private fun showDeleteLayout(holder: ViewHolder) {
// 显示删除按钮
val layoutParams = holder.itemView.layoutParams as ConstraintLayout.LayoutParams
layoutParams.width = holder.itemView.width - dpToPx(60)
holder.itemView.layoutParams = layoutParams
holder.itemView.findViewById<ConstraintLayout>(R.id.delete_layout).visibility = View.VISIBLE
}
private fun hideDeleteLayout(holder: ViewHolder) {
// 隐藏删除按钮
val layoutParams = holder.itemView.layoutParams as ConstraintLayout.LayoutParams
layoutParams.width = holder.itemView.width + dpToPx(60)
holder.itemView.layoutParams = layoutParams
holder.itemView.findViewById<ConstraintLayout>(R.id.delete_layout).visibility = View.GONE
}
private fun deleteItem(holder: ViewHolder) {
// 删除 item
list.toMutableList().removeAt(holder.adapterPosition)
notifyItemRemoved(holder.adapterPosition)
}
private fun dpToPx(dp: Int): Int {
val density = Resources.getSystem().displayMetrics.density
return (dp * density).toInt()
}
companion object {
private const val touchSlop = 10 // 滑动的最小距离
private const val clickTimeout = 200 // 点击的最大时间
private var xDown = 0f // 按下的 x 坐标
private var yDown = 0f // 按下的 y 坐标
private var timeDown = 0L // 按下的时间
}
}
```
3. 在 Activity 或 Fragment 中使用 RecyclerView 和 Adapter:
```
class MainActivity : AppCompatActivity() {
private lateinit var recyclerView: RecyclerView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
recyclerView = findViewById(R.id.recycler_view)
val list = listOf("Item 1", "Item 2", "Item 3", "Item 4", "Item 5")
recyclerView.adapter = MyAdapter(list)
recyclerView.layoutManager = LinearLayoutManager(this)
}
}
```
这样,你就可以实现一个带左滑删除功能的 RecyclerView 了。
阅读全文
相关推荐
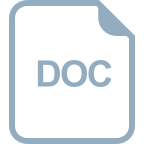
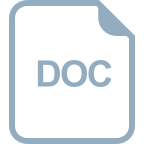
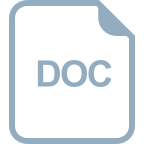






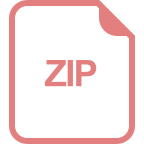








