用C语言写一段代码,从键盘上输入一个字符串,分别统计其中有多少个数字字符、英文字母、空格符,并打印输出结果。(要求:“统计字符串有多少个数字字符、英文字母、空格符”写成一个函数)
时间: 2023-03-06 11:16:28 浏览: 158
#include<stdio.h>
#include<string.h>void count_char(char *str)
{
int i;
int count_number = 0;
int count_letter = 0;
int count_space = 0; for(i=0;i<strlen(str);i++)
{
if(str[i]>='0' && str[i]<='9')
count_number++;
else if((str[i]>='a' && str[i]<='z') || (str[i]>='A' && str[i]<='Z'))
count_letter++;
else if(str[i]==' ')
count_space++;
} printf("字符串中数字字符有%d个,英文字母有%d个,空格符有%d个。\n", count_number, count_letter, count_space);
}int main()
{
char str[100];
printf("请输入一个字符串:");
gets(str);
count_char(str); return 0;
}
相关问题
用C语言代码写一个输入一个字符串,分别统计其中英文字母、数字、空格以及其他字符的个数。
以下是一个简单的 C 语言代码实现:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i, digits = 0, letters = 0, spaces = 0, others = 0;
printf("请输入一个字符串:\n");
gets(str);
for(i = 0; str[i] != '\0'; i++) {
if(isdigit(str[i])) {
digits++;
} else if(isalpha(str[i])) {
letters++;
} else if(str[i] == ' ') {
spaces++;
} else {
others++;
}
}
printf("数字个数:%d\n", digits);
printf("字母个数:%d\n", letters);
printf("空格个数:%d\n", spaces);
printf("其他字符个数:%d\n", others);
return 0;
}
```
代码中使用了一个 char 类型的数组来存储输入的字符串,然后使用一个 for 循环遍历字符串中的每一个字符,通过判断字符的类型(数字、字母、空格、其他字符)来分别统计它们的个数。最后输出统计结果。注意,这里使用了 gets() 函数来读取输入的字符串,但是这个函数存在安全问题,建议使用 fgets() 函数代替。
用C语言设计一个程序,从键盘输入一行字符,统计其中分别有多少大小写字母
这是两个不同的程序,但都可以实现统计输入字符串中大小写字母的个数的功能。下面是其中一个程序的解释:
```c
#include <stdio.h>
int main() {
int a=0,b=0,c=0,d=0;
char *p,i=0,s[100];
while((s[i]=getchar())!='\n') //一行!!!,所以结束标志为'\n'
{
i++;
}
p=&s[0];
while(*p!='\n') {
if((('A'<=*p)&&(*p<='Z'))||(('a'<=*p)&&(*p<='z'))) //大小写都要注意
a++;
else if(*p==' ')
b++;
else if(*p>='0'&&*p<='9')
c++;
else
d++;
p++; //这个别忘了加!
}
printf("英文字母,空格,数字,其他字符的个数分别为:%d %d %d %d\n",a,b,c,d);
return 0;
}
```
阅读全文
相关推荐
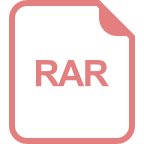
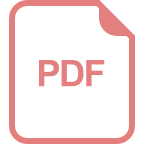













