帮我用java写一个UDP发文件的程序
时间: 2023-08-24 10:08:17 浏览: 80
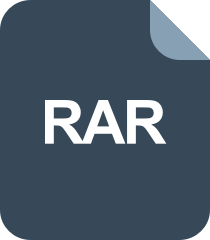
用UDP传送文件的程序
好的,以下是一个简单的UDP发送文件的Java程序:
```java
import java.io.*;
import java.net.*;
public class UDPSender {
public static void main(String[] args) {
DatagramSocket socket = null;
FileInputStream in = null;
try {
// 创建DatagramSocket对象
socket = new DatagramSocket();
// 读取文件内容
File file = new File("path/to/file");
in = new FileInputStream(file);
byte[] buffer = new byte[(int) file.length()];
in.read(buffer);
// 创建DatagramPacket对象
InetAddress address = InetAddress.getByName("接收方IP地址");
int port = 8888;
DatagramPacket packet = new DatagramPacket(buffer, buffer.length, address, port);
// 发送数据
socket.send(packet);
System.out.println("文件发送成功!");
} catch (IOException e) {
System.out.println("文件发送失败:" + e.getMessage());
} finally {
try {
if (in != null) {
in.close();
}
if (socket != null) {
socket.close();
}
} catch (IOException e) {
System.out.println("关闭资源失败:" + e.getMessage());
}
}
}
}
```
以上代码中需要替换的部分为:
- `"path/to/file"`:需要发送的文件路径。
- `"接收方IP地址"`:接收方的IP地址。
当接收方收到UDP数据包后,可以使用类似以下的代码将数据保存为文件:
```java
FileOutputStream out = new FileOutputStream("path/to/destination/file");
out.write(packet.getData());
out.close();
```
其中,`packet`为接收到的`DatagramPacket`对象。
阅读全文
相关推荐
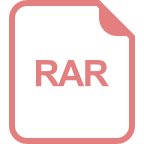
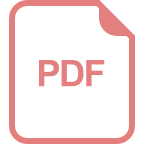
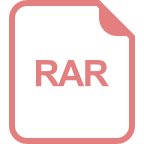
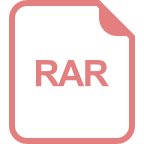
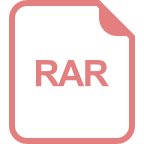
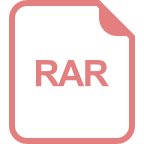
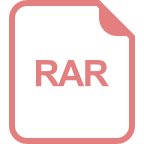
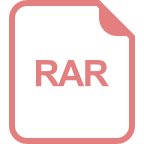
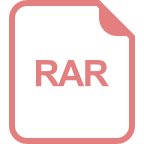
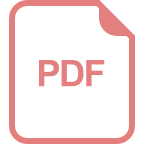
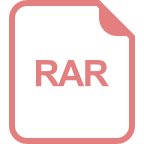
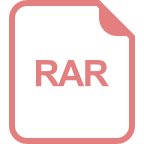
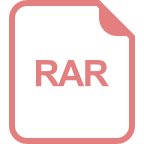
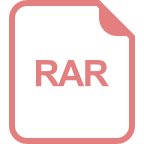
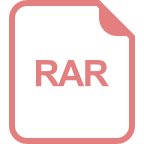
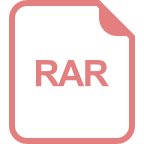