SVM网格搜索法怎么使用TensorFlow调用GPU python
时间: 2023-10-11 09:08:17 浏览: 160
要使用TensorFlow调用GPU进行SVM网格搜索,可以按照以下步骤进行:
1. 首先,确保已经安装了TensorFlow和相应的GPU驱动程序。
2. 在代码中导入TensorFlow和其他必要的库,例如numpy和sklearn等。
3. 使用sklearn的GridSearchCV方法来设置SVM的超参数网格搜索,具体方法如下:
```python
from sklearn.model_selection import GridSearchCV
from sklearn.svm import SVC
from sklearn.metrics import classification_report
import numpy as np
import tensorflow as tf
# 加载数据
X_train, y_train = load_data()
# 定义SVM模型
model = SVC(kernel='rbf')
# 定义要搜索的超参数
param_grid = {'C': [0.1, 1, 10], 'gamma': [0.1, 1, 10]}
# 使用GridSearchCV进行网格搜索
grid_search = GridSearchCV(model, param_grid, cv=5)
grid_search.fit(X_train, y_train)
# 输出最佳模型及其超参数
print("Best parameters: ", grid_search.best_params_)
print("Best score: ", grid_search.best_score_)
```
4. 使用TensorFlow的GPU加速特性,可以将数据转换为Numpy数组,然后使用`tf.data.Dataset.from_tensor_slices()`方法将其转换为TensorFlow数据集。具体方法如下:
```python
# 将数据转换为Numpy数组
X_train_numpy = np.array(X_train)
y_train_numpy = np.array(y_train)
# 将数据转换为TensorFlow数据集
train_dataset = tf.data.Dataset.from_tensor_slices((X_train_numpy, y_train_numpy))
```
5. 在训练SVM模型时,使用`tf.device()`方法指定使用的GPU设备,这样可以加速训练过程。具体方法如下:
```python
# 定义SVM模型
model = SVC(kernel='rbf', C=grid_search.best_params_['C'], gamma=grid_search.best_params_['gamma'])
# 使用GPU训练模型
with tf.device('/GPU:0'):
model.fit(train_dataset)
```
6. 在训练完成后,使用训练好的模型进行预测等任务。
阅读全文
相关推荐
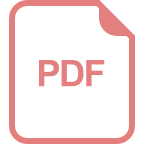


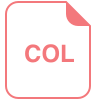
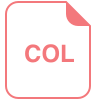
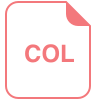
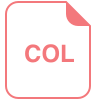
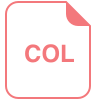
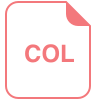
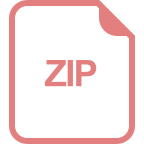