SVM怎么使用TensorFlow调用GPU进行网格搜索法,提升搜索效率 python
时间: 2023-10-11 09:08:17 浏览: 79
要使用TensorFlow调用GPU进行网格搜索法,可以按照以下步骤进行:
1. 安装TensorFlow-GPU版本和scikit-learn库。
2. 加载数据集并进行数据预处理。
3. 定义SVM模型和参数空间。
4. 使用scikit-learn库中的GridSearchCV函数进行网格搜索。
5. 将SVM模型和最佳参数应用于数据集并进行训练。
6. 使用TensorFlow-GPU版本进行模型评估和测试。
下面是一个示例代码:
```python
import numpy as np
from sklearn import datasets, svm
from sklearn.model_selection import GridSearchCV
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
import tensorflow as tf
# 加载数据集
iris = datasets.load_iris()
X = iris.data
y = iris.target
# 数据预处理
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 定义SVM模型和参数空间
svm_model = svm.SVC(kernel='rbf')
param_grid = {'C': [0.1, 1, 10, 100], 'gamma': [0.001, 0.01, 0.1, 1]}
# 使用GridSearchCV函数进行网格搜索
grid_search = GridSearchCV(svm_model, param_grid, cv=5, n_jobs=-1)
grid_search.fit(X_train, y_train)
# 输出最佳参数
print("Best parameters: ", grid_search.best_params_)
# 训练模型并进行评估
svm_model_best = svm.SVC(kernel='rbf', C=grid_search.best_params_['C'], gamma=grid_search.best_params_['gamma'])
svm_model_best.fit(X_train, y_train)
y_pred = svm_model_best.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy: ", accuracy)
# 使用TensorFlow-GPU版本进行模型评估和测试
with tf.device('/device:GPU:0'):
y_pred = svm_model_best.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy using GPU: ", accuracy)
```
在上面的代码中,我们使用了GridSearchCV函数进行网格搜索,n_jobs参数设置为-1,表示使用所有的CPU核心或GPU进行并行计算,提高搜索效率。在最后使用TensorFlow-GPU版本进行模型评估和测试时,我们使用了tf.device函数将计算设备设置为GPU,以提高计算速度。
相关推荐
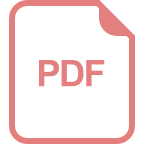














