java list stream().distinct() 去重使用
时间: 2023-10-24 09:15:13 浏览: 49
Java 中 List 接口提供了 stream() 方法,可以将 List 转换为 Stream,并在 Stream 上执行各种操作。其中,distinct() 方法用于去除 Stream 中的重复元素。
下面是示例代码:
```java
List<Integer> list = Arrays.asList(1, 2, 2, 3, 4, 4, 5);
List<Integer> distinctList = list.stream().distinct().collect(Collectors.toList());
System.out.println(distinctList); // 输出 [1, 2, 3, 4, 5]
```
在上述代码中,我们创建了一个包含重复元素的 List 对象,然后使用 stream() 方法将其转换为 Stream。接着,使用 distinct() 方法去重,并使用 collect(Collectors.toList()) 方法将 Stream 转换为 List 对象。
最后,打印输出去重后的 List 对象。
相关问题
list.stream.filter去重
list.stream.filter去重是Java 8中Stream API提供的一种操作,可以对一个List进行去重操作。具体实现方式是通过Stream API中的distinct()方法,该方法会返回一个去重后的Stream。使用filter()方法可以对Stream中的元素进行过滤,只保留符合条件的元素。因此,list.stream.filter去重的实现方式是先将List转换为Stream,然后使用distinct()方法进行去重,最后使用filter()方法对去重后的Stream进行过滤。
java list stream().distinct() 对象去重使用
如果要对一个 List 中的对象进行去重,可以使用 Java 8 中的 Stream API 和 distinct() 方法。
假设我们有一个 Person 类,它有两个属性 name 和 age:
```
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
现在我们有一个 List<Person>,我们想要按照 name 去重,可以使用以下代码:
```
List<Person> people = // 获取 List<Person> 对象
List<Person> uniquePeople = people.stream()
.distinct()
.collect(Collectors.toList());
```
需要注意的是,为了让 distinct() 方法正确去重,需要在 Person 类中实现 equals() 和 hashCode() 方法。如果没有实现,它会默认使用 Object 类中的 equals() 方法进行比较,这通常不是我们想要的。因此,Person 类应该像下面这样实现:
```
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person person = (Person) o;
return age == person.age &&
Objects.equals(name, person.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
```
这样,当我们使用 distinct() 方法时,它就会正确地根据 name 进行去重。
相关推荐
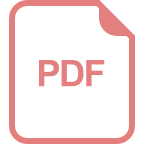










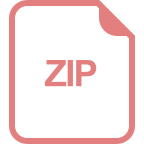
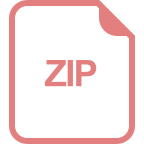
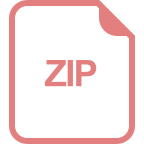
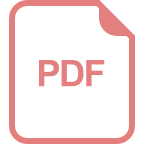