list.stream 指定元素去重
时间: 2023-10-05 16:08:29 浏览: 46
可以使用 Java 8 中的流(Stream)和 Lambda 表达式来实现指定元素去重。需要使用 distinct() 方法来去除重复元素,然后使用 filter() 方法指定需要去重的元素,最后使用 collect() 方法将结果收集到一个列表中。例如,以下代码可以去除列表中所有以字母 "a" 开头的重复元素:
```
List<String> list = Arrays.asList("apple", "banana", "avocado", "apricot", "banana", "cherry");
List<String> distinctList = list.stream()
.filter(str -> str.startsWith("a"))
.distinct()
.collect(Collectors.toList());
System.out.println(distinctList);
```
输出结果为:
```
[apple, avocado, apricot]
```
相关问题
list.stream 指定对象元素去重
可以使用 `distinct()` 方法来对 `Stream` 中的元素进行去重操作,例如:
```java
List<String> list = Arrays.asList("apple", "banana", "orange", "banana", "kiwi", "orange");
List<String> distinctList = list.stream().distinct().collect(Collectors.toList());
System.out.println(distinctList); // 输出结果为:[apple, banana, orange, kiwi]
```
这样就可以将 `list` 中重复的元素去掉,得到一个不含重复元素的新列表 `distinctList`。
.stream怎么去重
Java 8 中的 Stream 提供了多种去重方式,包括使用 distinct() 方法、collectingAndThen() 和 toCollection() 方法以及 filter() 方法。其中,distinct() 方法是最简单的一种方式,它可以去除 Stream 中重复的元素。collectingAndThen() 和 toCollection() 方法可以将 Stream 中的元素收集到一个集合中,并去除其中的重复元素。filter() 方法可以根据指定条件过滤掉 Stream 中的重复元素。
下面是具体的实现方法:
1. 使用 distinct() 方法
```java
List<Integer> list = Arrays.asList(1, 2, 3, 2, 4, 3, 5);
List<Integer> distinctList = list.stream().distinct().collect(Collectors.toList());
System.out.println(distinctList); // [1, 2, 3, 4, 5]
```
2. 使用 collectingAndThen() 和 toCollection() 方法
```java
List<User> userList = Arrays.asList(new User("Tom"), new User("Jerry"), new User("Tom"));
List<User> distinctUserList = userList.stream().collect(
Collectors.collectingAndThen(
Collectors.toCollection(() -> new TreeSet<>(Comparator.comparing(User::getName))),
ArrayList::new
)
);
System.out.println(distinctUserList); // [User{name='Jerry'}, User{name='Tom'}]
```
3. 使用 filter() 方法
```java
List<User> userList = Arrays.asList(new User("Tom"), new User("Jerry"), new User("Tom"));
List<User> distinctUserList = userList.stream().filter(
distinctByKey(User::getName)
).collect(Collectors.toList());
System.out.println(distinctUserList); // [User{name='Tom'}, User{name='Jerry'}]
public static <T> Predicate<T> distinctByKey(Function<? super T, Object> keyExtractor) {
Map<Object, Boolean> seen = new ConcurrentHashMap<>();
return t -> seen.putIfAbsent(keyExtractor.apply(t), Boolean.TRUE) == null;
}
```
阅读全文
相关推荐
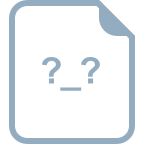
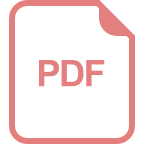













