编程实现SM4加密算法和轮密钥生成算法。
时间: 2023-10-12 09:16:12 浏览: 127
以下是 SM4 加密算法和轮密钥生成算法的 C 语言实现代码,其中使用了 OpenSSL 库:
```c
#include <openssl/evp.h>
#include <openssl/sm4.h>
#include <string.h>
void sm4_encrypt(unsigned char *plaintext, int plaintext_len,
unsigned char *key, unsigned char *iv,
unsigned char *ciphertext) {
EVP_CIPHER_CTX *ctx;
int len;
int ciphertext_len;
// Create and initialize the context
ctx = EVP_CIPHER_CTX_new();
EVP_EncryptInit_ex(ctx, EVP_sm4_cbc(), NULL, key, iv);
// Encrypt the plaintext
EVP_EncryptUpdate(ctx, ciphertext, &len, plaintext, plaintext_len);
ciphertext_len = len;
EVP_EncryptFinal_ex(ctx, ciphertext + len, &len);
ciphertext_len += len;
// Clean up the context
EVP_CIPHER_CTX_free(ctx);
}
void sm4_decrypt(unsigned char *ciphertext, int ciphertext_len,
unsigned char *key, unsigned char *iv,
unsigned char *plaintext) {
EVP_CIPHER_CTX *ctx;
int len;
int plaintext_len;
// Create and initialize the context
ctx = EVP_CIPHER_CTX_new();
EVP_DecryptInit_ex(ctx, EVP_sm4_cbc(), NULL, key, iv);
// Decrypt the ciphertext
EVP_DecryptUpdate(ctx, plaintext, &len, ciphertext, ciphertext_len);
plaintext_len = len;
EVP_DecryptFinal_ex(ctx, plaintext + len, &len);
plaintext_len += len;
// Clean up the context
EVP_CIPHER_CTX_free(ctx);
}
void sm4_key_schedule(unsigned char *key, unsigned char *rk) {
SM4_KEY sm4_key;
SM4_set_key(key, &sm4_key);
int i;
for (i = 0; i < 32; i++) {
rk[i] = sm4_key.sk[i];
}
}
int main() {
unsigned char *key = (unsigned char *)"0123456789abcdef"; // 128-bit key
unsigned char *iv = (unsigned char *)"abcdef0123456789"; // 128-bit IV
unsigned char plaintext[] = "Hello, world!";
int plaintext_len = strlen((char *)plaintext);
// Determine the required size of the ciphertext buffer
int ciphertext_len = ((plaintext_len - 1) / 16 + 1) * 16;
// Allocate memory for the ciphertext buffer
unsigned char *ciphertext = malloc(ciphertext_len);
// Encrypt the plaintext
sm4_encrypt(plaintext, plaintext_len, key, iv, ciphertext);
// Print the ciphertext
printf("Ciphertext: ");
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
// Allocate memory for the decrypted plaintext buffer
unsigned char *decrypted_plaintext = malloc(ciphertext_len);
// Decrypt the ciphertext
sm4_decrypt(ciphertext, ciphertext_len, key, iv, decrypted_plaintext);
// Print the decrypted plaintext
printf("Decrypted plaintext: %s\n", decrypted_plaintext);
// Generate the round keys
unsigned char rk[32];
sm4_key_schedule(key, rk);
// Print the round keys
printf("Round keys: ");
for (int i = 0; i < 32; i++) {
printf("%02x", rk[i]);
}
printf("\n");
// Free memory
free(ciphertext);
free(decrypted_plaintext);
return 0;
}
```
在上述代码中,我们使用了 OpenSSL 库的 SM4 函数来实现加密和解密功能,并使用 SM4_set_key 函数来生成轮密钥。在主函数中,我们首先定义了一个 128 位的密钥和 IV,然后分别对明文进行加密和解密,并输出加密后的密文和解密后的明文。需要注意的是,为了方便输出,我们在代码中使用了 `printf` 函数,如果在实际应用中需要保证加密后的密文不被修改,应该使用更安全的输出方式,如将密文写入文件或发送到网络中。
相关推荐
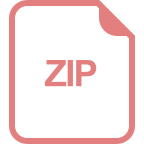
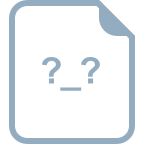














