rinex o文件读取
时间: 2023-06-07 14:01:49 浏览: 517
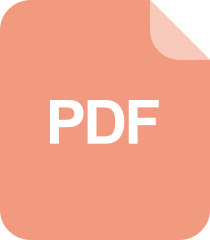
GPS观测O文件头部分rinex格式-详细说明[定义].pdf
RINEX (Receiver Independent Exchange Format) O文件是卫星导航接收机数据的标准格式。RINEX O文件记录卫星导航接收机观测到的卫星信号数据,包括卫星位置、观测时间、卫星信号的伪距和相位等。这些数据可以通过专业软件进行处理,例如进行定位和导航。在读取RINEX O文件时需要注意以下几点。
首先,需要确认RINEX O文件的版本。RINEX O文件有不同的版本,不同版本的文件可能使用不同的格式来记录数据,因此读取时需要确认文件版本。
其次,需要了解RINEX O文件中记录的数据类型。RINEX O文件中记录的数据类型包括卫星星历数据、观测数据等,需要根据实际需要读取相应的数据类型。
在读取时,需要使用专业软件对RINEX O文件进行解码。常用的软件包括RTKLIB、TEQC等。
最后,在读取RINEX O文件时需要注意数据的精度和稳定性。由于卫星信号受多种因素影响,包括天气、地形等因素,因此读取到的数据可能存在误差。在后续处理中需要对数据进行筛选和修正,确保数据的精度和稳定性。
阅读全文
相关推荐
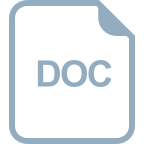
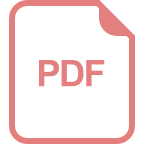












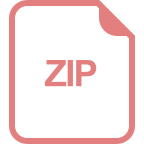


