#include <iostream> using namespace std; class Time { public: int hour; // 小时 int minute; // 分钟 Time(int h=0, int m=0) { hour = h; minute = m; } void show() { cout << hour << ":" << minute << endl; } // write your code here...... //重载运算符+,使得可以进行两个时间类对象的相加,返回相加,或相减的结果。 //两个时间对象的相加,注意,分钟+分钟,小时+小时,分钟大于60,小时大于24怎么办? }; /* int main() { int h, m; cin >> h >> m; Time t1(h, m); Time t2(10, 20); Time t3 = t1 + t2; t3.show(); return 0; }*/
时间: 2023-11-28 16:50:03 浏览: 68
在上述代码中,需要重载运算符+,使得可以进行两个时间类对象的相加,返回相加或相减的结果。
下面是具体的实现方法:
```c++
Time operator+(const Time& t1, const Time& t2) {
Time t;
t.hour = t1.hour + t2.hour;
t.minute = t1.minute + t2.minute;
if (t.minute >= 60) {
t.hour += t.minute / 60;
t.minute %= 60;
}
if (t.hour >= 24) {
t.hour %= 24;
}
return t;
}
Time operator-(const Time& t1, const Time& t2) {
Time t;
t.hour = t1.hour - t2.hour;
t.minute = t1.minute - t2.minute;
if (t.minute < 0) {
t.hour -= 1;
t.minute += 60;
}
if (t.hour < 0) {
t.hour += 24;
}
return t;
}
```
在这里,我们定义了两个运算符函数,一个是加法运算符+,一个是减法运算符-。这两个函数的参数都是两个常量引用,分别表示要进行运算的两个时间对象。
在加法运算符+中,我们先创建一个新的时间对象t,并将t1和t2的小时数和分钟数相加赋值给t,然后进行进位操作。如果t的分钟数大于等于60,则将它们转换为小时数,同时将t的分钟数修改为不足60的余数。如果t的小时数大于等于24,则将它们修改为不足24的余数。
在减法运算符-中,我们同样先创建一个新的时间对象t,并将t1和t2的小时数和分钟数相减赋值给t,然后进行借位操作。如果t的分钟数小于0,则将它们转换为小时数,同时将t的分钟数修改为不足60的余数。如果t的小时数小于0,则将它们修改为不足24的余数。
最后,我们可以在主函数中测试这两个运算符函数:
```c++
int main() {
int h, m;
cin >> h >> m;
Time t1(h, m);
Time t2(10, 20);
Time t3 = t1 + t2;
t3.show();
Time t4 = t1 - t2;
t4.show();
return 0;
}
```
这里我们先输入一个时间t1,然后创建另一个时间t2,并对它们进行加减法运算,最后输出结果。
相关推荐
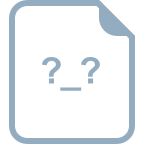













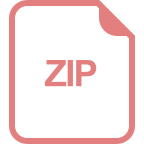