库存管理系统java+sql代码
时间: 2023-12-13 14:04:17 浏览: 67
很抱歉,由于库存管理系统的代码量较大,无法在此处一一展示。下面提供一个简单的示例,希望能对您有所帮助。
1. Java代码示例:
```
// 商品类
public class Product {
private int id; // 商品编号
private String name; // 商品名称
private double price; // 商品价格
private int quantity; // 商品数量
// 构造方法
public Product(int id, String name, double price, int quantity) {
this.id = id;
this.name = name;
this.price = price;
this.quantity = quantity;
}
// getter 和 setter 方法
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
// 数据库操作类
public class ProductDao {
// 声明数据库连接对象
private Connection conn;
// 构造方法
public ProductDao() {
// 获取数据库连接
conn = DBUtil.getConnection();
}
// 查询商品列表
public List<Product> getProductList() {
List<Product> productList = new ArrayList<>();
try {
// 查询商品列表的 SQL 语句
String sql = "SELECT * FROM product";
// 创建 PreparedStatement 对象
PreparedStatement pstmt = conn.prepareStatement(sql);
// 执行 SQL 查询语句
ResultSet rs = pstmt.executeQuery();
// 遍历查询结果集,将数据封装到 Product 对象中
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
double price = rs.getDouble("price");
int quantity = rs.getInt("quantity");
Product product = new Product(id, name, price, quantity);
productList.add(product);
}
// 关闭 ResultSet 对象和 PreparedStatement 对象
rs.close();
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
return productList;
}
// 添加商品
public void addProduct(Product product) {
try {
// 添加商品的 SQL 语句
String sql = "INSERT INTO product (id, name, price, quantity) VALUES (?, ?, ?, ?)";
// 创建 PreparedStatement 对象
PreparedStatement pstmt = conn.prepareStatement(sql);
// 设置 SQL 语句中的参数
pstmt.setInt(1, product.getId());
pstmt.setString(2, product.getName());
pstmt.setDouble(3, product.getPrice());
pstmt.setInt(4, product.getQuantity());
// 执行 SQL 添加语句
pstmt.executeUpdate();
// 关闭 PreparedStatement 对象
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 更新商品信息
public void updateProduct(Product product) {
try {
// 更新商品信息的 SQL 语句
String sql = "UPDATE product SET name=?, price=?, quantity=? WHERE id=?";
// 创建 PreparedStatement 对象
PreparedStatement pstmt = conn.prepareStatement(sql);
// 设置 SQL 语句中的参数
pstmt.setString(1, product.getName());
pstmt.setDouble(2, product.getPrice());
pstmt.setInt(3, product.getQuantity());
pstmt.setInt(4, product.getId());
// 执行 SQL 更新语句
pstmt.executeUpdate();
// 关闭 PreparedStatement 对象
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
// 删除商品
public void deleteProduct(int id) {
try {
// 删除商品的 SQL 语句
String sql = "DELETE FROM product WHERE id=?";
// 创建 PreparedStatement 对象
PreparedStatement pstmt = conn.prepareStatement(sql);
// 设置 SQL 语句中的参数
pstmt.setInt(1, id);
// 执行 SQL 删除语句
pstmt.executeUpdate();
// 关闭 PreparedStatement 对象
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
// 数据库连接类
public class DBUtil {
// 数据库连接信息
private static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
private static final String DB_URL = "jdbc:mysql://localhost:3306/inventory";
private static final String USER = "root";
private static final String PASSWORD = "123456";
// 获取数据库连接
public static Connection getConnection() {
Connection conn = null;
try {
// 注册 JDBC 驱动
Class.forName(JDBC_DRIVER);
// 打开数据库连接
conn = DriverManager.getConnection(DB_URL, USER, PASSWORD);
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
return conn;
}
}
```
2. SQL代码示例:
```
-- 创建商品表
CREATE TABLE product (
id INT PRIMARY KEY NOT NULL,
name VARCHAR(50) NOT NULL,
price DOUBLE NOT NULL,
quantity INT NOT NULL
);
-- 插入商品数据
INSERT INTO product (id, name, price, quantity) VALUES (1, 'Apple', 5.99, 100);
INSERT INTO product (id, name, price, quantity) VALUES (2, 'Banana', 3.99, 200);
INSERT INTO product (id, name, price, quantity) VALUES (3, 'Orange', 4.99, 150);
-- 查询商品列表
SELECT * FROM product;
-- 添加商品
INSERT INTO product (id, name, price, quantity) VALUES (4, 'Grape', 7.99, 50);
-- 更新商品信息
UPDATE product SET name='Grapefruit', price=6.99, quantity=80 WHERE id=4;
-- 删除商品
DELETE FROM product WHERE id=4;
```
相关推荐
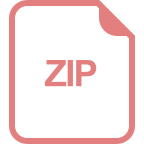
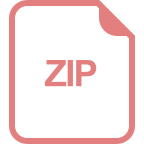
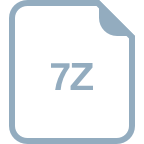
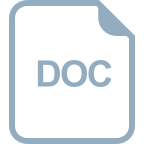
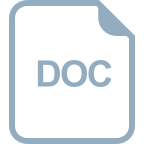
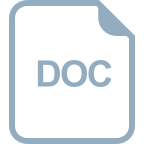
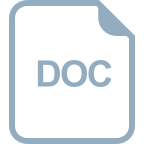
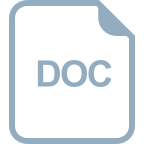









