用python制作扫雷程序
时间: 2023-12-27 14:04:47 浏览: 84
以下是一个基本的扫雷程序示例,使用Python语言编写:
```python
import random
class Minesweeper:
def __init__(self, width, height, num_mines):
self.width = width
self.height = height
self.num_mines = num_mines
self.board = [[0 for i in range(width)] for j in range(height)]
self.mines = set()
def generate_board(self):
# Place mines randomly
while len(self.mines) < self.num_mines:
x = random.randint(0, self.width - 1)
y = random.randint(0, self.height - 1)
self.mines.add((x, y))
# Fill in numbers around mines
for x, y in self.mines:
self.board[y][x] = -1
for i in range(-1, 2):
for j in range(-1, 2):
if (x + i) >= 0 and (x + i) < self.width and (y + j) >= 0 and (y + j) < self.height and self.board[y+j][x+i] != -1:
self.board[y+j][x+i] += 1
def show_board(self):
for row in self.board:
for cell in row:
if cell == -1:
print('*', end='')
else:
print(cell, end='')
print()
def play(self):
self.generate_board()
self.show_board()
game = Minesweeper(10, 10, 10)
game.play()
```
该程序创建了一个名为“Minesweeper”的类,其构造函数接受三个参数:游戏板的宽度、高度和地雷数量。 `generate_board`方法随机放置地雷并在地雷周围的方格中填写数字。 `show_board`方法显示游戏板。 `play`方法调用 `generate_board`和 `show_board`方法以开始游戏。
该程序可以通过对类进行扩展来实现其他功能,例如让用户输入坐标来揭示方格,或者添加计时器以记录游戏时间。
阅读全文
相关推荐
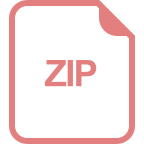
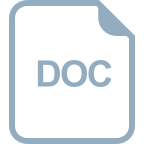


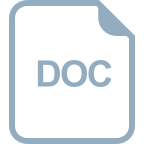

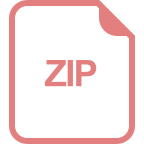
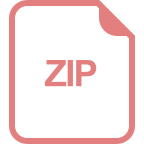
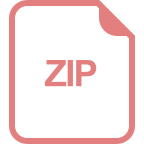
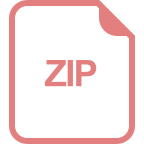
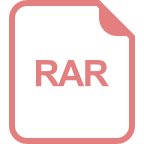
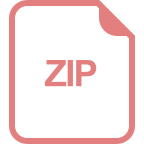
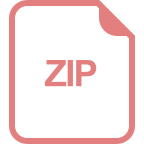
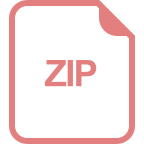
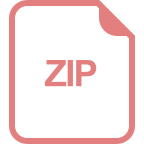
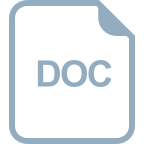