基于java对操作系统进程管理问题读者与写者编写代码测试且注释
时间: 2023-12-18 18:05:50 浏览: 31
好的,以下是基于 Java 的读者与写者问题的代码,包含注释。
```
// 定义一个共享资源类
class SharedResource {
private int data;
private boolean writerActive;
// 读取数据方法
public synchronized int readData() {
// 如果还有写者在写,则等待
while (writerActive) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// 读取数据
return data;
}
// 写入数据方法
public synchronized void writeData(int newData) {
// 标记写者正在写
writerActive = true;
// 写入数据
data = newData;
// 标记写者已经结束写入
writerActive = false;
// 唤醒所有等待线程
notifyAll();
}
}
// 定义一个读者类
class Reader extends Thread {
private SharedResource resource;
public Reader(String name, SharedResource resource) {
super(name);
this.resource = resource;
}
@Override
public void run() {
// 循环读取数据
while (true) {
// 读取数据
int data = resource.readData();
// 输出读取结果
System.out.println(getName() + " read data: " + data);
// 等待 1 秒
try {
sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
// 定义一个写者类
class Writer extends Thread {
private SharedResource resource;
public Writer(String name, SharedResource resource) {
super(name);
this.resource = resource;
}
@Override
public void run() {
// 循环写入数据
while (true) {
// 生成随机数
int data = (int) (Math.random() * 100);
// 写入数据
resource.writeData(data);
// 输出写入结果
System.out.println(getName() + " write data: " + data);
// 等待 1 秒
try {
sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
// 测试代码
public class Test {
public static void main(String[] args) {
// 创建共享资源对象
SharedResource resource = new SharedResource();
// 创建 5 个读者线程
for (int i = 1; i <= 5; i++) {
Reader reader = new Reader("Reader-" + i, resource);
reader.start();
}
// 创建 2 个写者线程
for (int i = 1; i <= 2; i++) {
Writer writer = new Writer("Writer-" + i, resource);
writer.start();
}
}
}
```
以上代码实现了读者与写者问题的经典解法,使用了 Java 中的 synchronized 关键字来实现线程同步。其中,SharedResource 类表示共享资源,包含了读取数据和写入数据的方法,使用了 synchronized 关键字来保证方法的原子性和线程同步。Reader 类表示读者线程,循环读取数据,并输出读取结果;Writer 类表示写者线程,循环生成随机数并写入共享资源,并输出写入结果。Test 类为测试代码,创建了 5 个读者线程和 2 个写者线程,并启动它们。
相关推荐
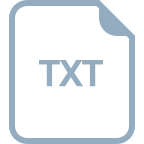








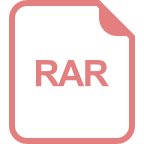
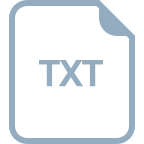
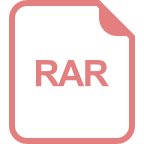
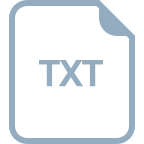
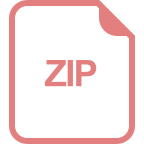