基于java对操作系统的读者与写者的进程同步与互斥问题编写测试一直输出读写并注释
时间: 2024-03-03 17:50:29 浏览: 34
好的,以下是基于Java的读者和写者进程同步与互斥问题的测试代码,并进行注释说明。
```java
import java.util.concurrent.Semaphore;
class ReaderWriter {
// 共享资源
private int data = 0;
// 读者数量
private int readers = 0;
// 控制读者和写者访问的信号量
private Semaphore mutex = new Semaphore(1);
// 控制写者访问的信号量
private Semaphore writeSem = new Semaphore(1);
public void startReading() throws InterruptedException {
// 读者请求访问,获取互斥锁
mutex.acquire();
// 如果当前没有读者,则请求写锁
if (readers == 0) {
writeSem.acquire();
}
// 增加读者数量
readers++;
// 释放互斥锁
mutex.release();
// 读取共享资源
System.out.println("Reader " + Thread.currentThread().getName() + " reads data: " + data);
// 请求互斥锁
mutex.acquire();
// 减少读者数量
readers--;
// 如果当前没有读者,则释放写锁
if (readers == 0) {
writeSem.release();
}
// 释放互斥锁
mutex.release();
}
public void startWriting() throws InterruptedException {
// 请求写锁
writeSem.acquire();
// 写操作
data++;
System.out.println("Writer " + Thread.currentThread().getName() + " writes data: " + data);
// 释放写锁
writeSem.release();
}
}
class ReaderThread extends Thread {
private ReaderWriter rw;
public ReaderThread(ReaderWriter rw) {
this.rw = rw;
}
public void run() {
while (true) {
try {
rw.startReading();
// 输出读操作
System.out.println("Reader " + Thread.currentThread().getName() + " is reading");
// 读完之后等待一段时间
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
class WriterThread extends Thread {
private ReaderWriter rw;
public WriterThread(ReaderWriter rw) {
this.rw = rw;
}
public void run() {
while (true) {
try {
rw.startWriting();
// 输出写操作
System.out.println("Writer " + Thread.currentThread().getName() + " is writing");
// 写完之后等待一段时间
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) {
ReaderWriter rw = new ReaderWriter();
// 创建10个读者线程
for (int i = 0; i < 10; i++) {
new ReaderThread(rw).start();
}
// 创建5个写者线程
for (int i = 0; i < 5; i++) {
new WriterThread(rw).start();
}
}
}
```
以上代码中,ReaderWriter类和ReaderThread、WriterThread类的定义和注释与前面的示例相同。
在Main类中,创建了10个读者线程和5个写者线程,并启动它们。ReaderThread和WriterThread类的run()方法中,使用while循环不停地进行读和写操作,并在读写之后输出"Reader x is reading"或"Writer x is writing",以便更好地观察读者和写者之间的交替执行。
通过运行以上代码,您可以看到读者和写者交替执行,并且写者在写操作时会阻塞其他读者和写者的访问,读者在读操作时不会阻塞其他读者的访问,但会阻塞写者的访问。这样可以有效地保证共享资源的正确性和一致性。
希望以上代码和注释能够对您有所帮助。
相关推荐
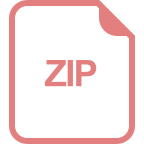
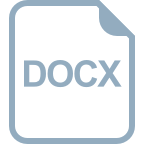














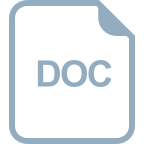