8266控制RGB灯带,编写一个声控灯的代码
时间: 2024-03-08 21:47:46 浏览: 19
以下是一个简单的Arduino代码示例,使用8266控制RGB灯带制作声控灯效果:
```arduino
#include <ESP8266WiFi.h>
#include <ArduinoFFT.h>
// WiFi网络设置
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// RGB灯带引脚设置
#define RED_PIN D5
#define GREEN_PIN D6
#define BLUE_PIN D7
// FFT设置
const int SAMPLES = 1024;
const int SAMPLING_FREQUENCY = 8000;
const int LED_THRESHOLD = 5000;
// 创建FFT对象
ArduinoFFT FFT = ArduinoFFT();
void setup() {
pinMode(RED_PIN, OUTPUT);
pinMode(GREEN_PIN, OUTPUT);
pinMode(BLUE_PIN, OUTPUT);
// 连接WiFi网络
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("WiFi connected");
}
void loop() {
// 读取声音信号
double signal[SAMPLES];
for (int i = 0; i < SAMPLES; i++) {
signal[i] = analogRead(A0);
}
// 进行FFT变换
double vReal[SAMPLES];
double vImag[SAMPLES];
for (int i = 0; i < SAMPLES; i++) {
vReal[i] = signal[i];
vImag[i] = 0;
}
FFT.Windowing(vReal, SAMPLES, FFT_WIN_TYP_HAMMING, FFT_FORWARD);
FFT.Compute(vReal, vImag, SAMPLES, FFT_FORWARD);
FFT.ComplexToMagnitude(vReal, vImag, SAMPLES);
// 获取频率最大的值
double maxVal = 0;
int maxIdx = 0;
for (int i = 2; i < (SAMPLES / 2); i++) {
if (vReal[i] > maxVal) {
maxVal = vReal[i];
maxIdx = i;
}
}
// 根据频率值控制灯光
if (maxVal > LED_THRESHOLD) {
if (maxIdx < 2000) {
setColor(255, 0, 0); // 红色
} else if (maxIdx < 4000) {
setColor(0, 255, 0); // 绿色
} else {
setColor(0, 0, 255); // 蓝色
}
} else {
setColor(0, 0, 0); // 灭灯
}
// 等待100毫秒
delay(100);
}
// 设置RGB灯带颜色
void setColor(int red, int green, int blue) {
analogWrite(RED_PIN, red);
analogWrite(GREEN_PIN, green);
analogWrite(BLUE_PIN, blue);
}
```
该示例使用ESP8266连接WiFi网络,并在循环中读取声音信号,并进行FFT变换。根据频率最大的值,控制RGB灯带的颜色。在该示例中,灯带的引脚分别连接到D5、D6和D7引脚,声音传感器连接到A0引脚。你可以根据你的实际连接方式进行修改。
相关推荐
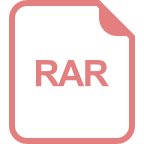














