怎么可以将所有的tensors全部放到cuda:1上运行的代码
时间: 2024-03-03 11:53:55 浏览: 218
您可以通过以下代码将所有的tensors全部放到cuda:1上运行:
```python
import torch
# 检查cuda是否可用
if torch.cuda.is_available():
# 设置默认设备为cuda:1
torch.cuda.set_device(1)
# 遍历所有tensors,将其移到cuda:1上
for name, param in model.named_parameters():
if param.requires_grad:
param.data = param.data.cuda()
```
这段代码会检查cuda是否可用,如果可用则将默认设备设置为cuda:1,并且遍历所有的tensors将其移到cuda:1上。需要注意的是,这段代码只会将requires_grad为True的tensors移到cuda:1上。如果某个tensor的requires_grad为False,则需要手动将其移到cuda:1上。
相关问题
RuntimeError: Expected all tensors to be on the same device, but found at least two devices cuda:0 and cuda:1
这个错误是由于在代码中使用了不同的设备导致的。具体来说,这个错误是因为在同一个操作中使用了在不同设备上的张量。要解决这个问题,你需要确保所有的张量都在同一个设备上。可以使用`.to(device)`方法将所有的张量转移到同一个设备上。
下面是一个示例代码,演示了如何解决这个错误:
```python
import torch
device = torch.device('cuda:0') # 指定设备
x = torch.tensor([1, 2, 3]).to(device) # 将张量 x 移到设备上
y = torch.tensor([4, 5, 6]).to(device) # 将张量 y 移到设备上
z = x + y # 这里的操作会在同一个设备上进行,不会出现错误
print(z)
```
RuntimeError: Expected all tensors to be on the same device, but found at least two devices, cuda:0 and cuda:1.
RuntimeError: "Expected all tensors to be on the same device" 这种错误通常发生在PyTorch等深度学习框架中,当你尝试对位于不同设备(如GPU)的张量(tensor)执行操作时。在并行计算或分布式训练中,所有数据和计算需要在同一设备上协调才能顺利进行。这提示你在某个操作中可能忘记将所有的张量移动到同一GPU,或者没有正确地配置好模型和数据加载器的设备归属。
解决这个问题,你需要检查以下几个方面:
1. 确保所有需要运算的张量都在同一个GPU(如cuda:0或cuda:1)上。
2. 使用`.to(device)` 或 `.move()` 将张量移动到正确的设备。
3. 如果你正在使用DataParallel、DistributedDataParallel这样的并行模块,确保它们正确配置了设备和相应的数据分配策略。
阅读全文
相关推荐
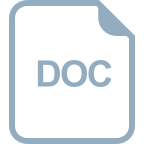
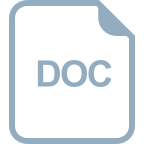
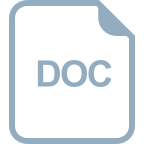


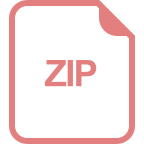








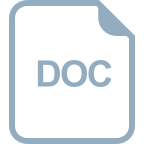
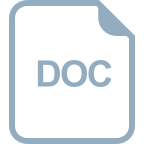