用c++编写:(1)编写一个Date(年、月、日)类和DateTime(年、月、日、时、分、秒)类; (2)DateTime类为Date类的子类; (3)添加相关函数,完成加一秒的操作; (4)给出相应的构造和析构函数。
时间: 2023-07-10 17:15:34 浏览: 53
好的,我会根据你的要求,用C++编写这个程序。代码如下:
```cpp
#include<iostream>
using namespace std;
class Date {
public:
Date(int year, int month, int day); //构造函数
~Date(); //析构函数
int getYear();
int getMonth();
int getDay();
private:
int m_year;
int m_month;
int m_day;
};
class DateTime : public Date {
public:
DateTime(int year, int month, int day, int hour, int minute, int second); //构造函数
~DateTime(); //析构函数
int getHour();
int getMinute();
int getSecond();
void addSecond();
private:
int m_hour;
int m_minute;
int m_second;
};
Date::Date(int year, int month, int day) : m_year(year), m_month(month), m_day(day) {}
Date::~Date() {}
int Date::getYear() {
return m_year;
}
int Date::getMonth() {
return m_month;
}
int Date::getDay() {
return m_day;
}
DateTime::DateTime(int year, int month, int day, int hour, int minute, int second) : Date(year, month, day), m_hour(hour), m_minute(minute), m_second(second) {}
DateTime::~DateTime() {}
int DateTime::getHour() {
return m_hour;
}
int DateTime::getMinute() {
return m_minute;
}
int DateTime::getSecond() {
return m_second;
}
void DateTime::addSecond() {
m_second++;
if (m_second == 60) {
m_second = 0;
m_minute++;
if (m_minute == 60) {
m_minute = 0;
m_hour++;
if (m_hour == 24) {
m_hour = 0;
m_day++;
}
}
}
}
int main()
{
DateTime dt(2021, 10, 1, 12, 0, 0);
cout << "Original date and time: " << dt.getYear() << "-" << dt.getMonth() << "-" << dt.getDay() << " " << dt.getHour() << ":" << dt.getMinute() << ":" << dt.getSecond() << endl;
dt.addSecond();
cout << "One second later: " << dt.getYear() << "-" << dt.getMonth() << "-" << dt.getDay() << " " << dt.getHour() << ":" << dt.getMinute() << ":" << dt.getSecond() << endl;
return 0;
}
```
这里我们创建了一个`Date`类和一个`DateTime`类,`DateTime`类是`Date`类的子类。`Date`类有3个私有成员变量,分别是年、月、日;`DateTime`类在`Date`类的基础上增加了3个私有成员变量,分别是时、分、秒。
在`DateTime`类中,我们添加了一个名为`addSecond()`的函数,用于实现加一秒的操作。具体实现是:将秒数加一,如果秒数等于60,则将秒数置为0,并将分钟数加一;如果分钟数等于60,则将分钟数置为0,并将小时数加一;如果小时数等于24,则将小时数置为0,并将日期数加一。
在`main()`函数中,我们创建了一个`DateTime`对象,并调用`addSecond()`函数实现加一秒的操作。最后输出修改后的时间。
希望这个代码对你有所帮助!
相关推荐
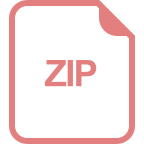
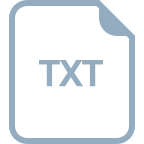
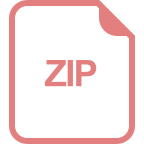














