简单继承 (1)编写一个Date(年、月、日)类和DateTime(年、月、日、时、分、秒)类; (2)DateTime类为Date类的子类; (3)添加相关函数,完成加一秒的操作; (4)给出相应的构造和析构函数。
时间: 2023-07-10 19:14:21 浏览: 39
好的,这是一个简单的继承问题,我可以帮你解决。
以下是Date类的实现:
```c++
class Date {
public:
Date(int year, int month, int day);
~Date();
void printDate();
protected:
int m_year;
int m_month;
int m_day;
};
Date::Date(int year, int month, int day)
: m_year(year), m_month(month), m_day(day) {}
Date::~Date() {}
void Date::printDate() {
std::cout << m_year << "-" << m_month << "-" << m_day << std::endl;
}
```
以下是DateTime类的实现:
```c++
class DateTime : public Date {
public:
DateTime(int year, int month, int day, int hour, int minute, int second);
~DateTime();
void printDateTime();
void addSecond();
private:
int m_hour;
int m_minute;
int m_second;
};
DateTime::DateTime(int year, int month, int day, int hour, int minute, int second)
: Date(year, month, day), m_hour(hour), m_minute(minute), m_second(second) {}
DateTime::~DateTime() {}
void DateTime::printDateTime() {
std::cout << m_year << "-" << m_month << "-" << m_day << " " << m_hour << ":" << m_minute << ":" << m_second << std::endl;
}
void DateTime::addSecond() {
m_second++;
if (m_second >= 60) {
m_second = 0;
m_minute++;
if (m_minute >= 60) {
m_minute = 0;
m_hour++;
if (m_hour >= 24) {
m_hour = 0;
m_day++;
}
}
}
}
```
在这里,DateTime类继承自Date类,因此我们可以使用Date类的成员变量和成员函数,也可以添加自己的成员变量和成员函数。addSecond()是我们添加的函数,它可以将DateTime对象的时间加一秒。
以下是构造和析构函数的实现:
```c++
Date::Date(int year, int month, int day)
: m_year(year), m_month(month), m_day(day) {}
Date::~Date() {}
DateTime::DateTime(int year, int month, int day, int hour, int minute, int second)
: Date(year, month, day), m_hour(hour), m_minute(minute), m_second(second) {}
DateTime::~DateTime() {}
```
这里我们只是简单地实现了构造和析构函数,没有做任何特殊的事情。
现在你可以创建一个DateTime对象并调用它的函数,例如:
```c++
DateTime myDateTime(2021, 9, 28, 8, 30, 0);
myDateTime.printDateTime(); // 输出:2021-9-28 8:30:0
myDateTime.addSecond();
myDateTime.printDateTime(); // 输出:2021-9-28 8:30:1
```
希望这个例子能帮到你!
相关推荐
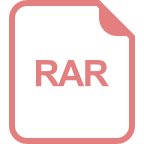
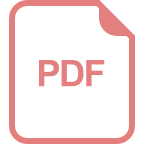
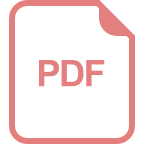









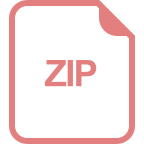
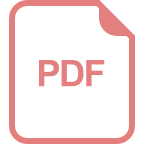
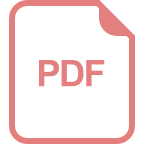
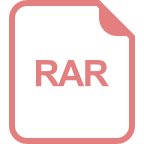
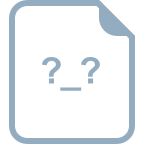