工资支付系统:为某公司编写一个工资支付系统,用于计算某一类员工的月薪。该公司共有四类员工:领固定月薪的(SalariedEmployee);计时取酬的(HourlyEmployee,如果一月工时超过160小时,则还需对额外的工时支付加班费);按销售额提成(CommissionEmployee)的和带底薪并按销售额提成的(BasePlusCommissionEmployee),其继承层次结构如下所示。已知每类员工均有表示员工工号、姓名和出生年月的属性,和用于计算员工月薪的方法。创建一个Employee变量数组,保存Employee类层次结构中每个具体类对象的引用,对每个Employee显示其工号、姓名、出生年月和月收入,如果当月是Employee的生日所在的月份,则还另发给他100月作为红包。
时间: 2024-02-17 11:02:18 浏览: 72
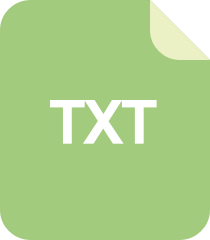
公司人事管理系统C++

好的,这是一个非常具体的问题,我会尽力回答。根据你的描述,我可以提供以下代码实现:
```python
import datetime
class Employee:
def __init__(self, emp_id, name, birthdate):
self.emp_id = emp_id
self.name = name
self.birthdate = birthdate
def get_monthly_salary(self):
pass
def get_bonus(self):
today = datetime.date.today()
if today.month == self.birthdate.month:
return 100
else:
return 0
class SalariedEmployee(Employee):
def __init__(self, emp_id, name, birthdate, monthly_salary):
super().__init__(emp_id, name, birthdate)
self.monthly_salary = monthly_salary
def get_monthly_salary(self):
return self.monthly_salary + self.get_bonus()
class HourlyEmployee(Employee):
def __init__(self, emp_id, name, birthdate, hourly_rate, hours_worked):
super().__init__(emp_id, name, birthdate)
self.hourly_rate = hourly_rate
self.hours_worked = hours_worked
def get_monthly_salary(self):
if self.hours_worked > 160:
overtime_pay = (self.hours_worked - 160) * (self.hourly_rate * 1.5)
monthly_salary = (160 * self.hourly_rate) + overtime_pay
else:
monthly_salary = self.hours_worked * self.hourly_rate
return monthly_salary + self.get_bonus()
class CommissionEmployee(Employee):
def __init__(self, emp_id, name, birthdate, commission_rate, sales):
super().__init__(emp_id, name, birthdate)
self.commission_rate = commission_rate
self.sales = sales
def get_monthly_salary(self):
return self.sales * self.commission_rate + self.get_bonus()
class BasePlusCommissionEmployee(CommissionEmployee):
def __init__(self, emp_id, name, birthdate, commission_rate, sales, base_salary):
super().__init__(emp_id, name, birthdate, commission_rate, sales)
self.base_salary = base_salary
def get_monthly_salary(self):
return self.base_salary + super().get_monthly_salary()
employees = [
SalariedEmployee(1, '张三', datetime.date(1980, 1, 1), 10000),
HourlyEmployee(2, '李四', datetime.date(1990, 2, 2), 50, 180),
CommissionEmployee(3, '王五', datetime.date(1985, 3, 3), 0.1, 50000),
BasePlusCommissionEmployee(4, '赵六', datetime.date(1995, 4, 4), 0.1, 40000, 5000)
]
for emp in employees:
print('工号:', emp.emp_id)
print('姓名:', emp.name)
print('出生年月:', emp.birthdate)
print('月收入:', emp.get_monthly_salary())
print('红包:', emp.get_bonus())
print('----------------------')
```
这段代码中,我们定义了一个抽象类 `Employee`,它有一个 `get_monthly_salary` 方法用于计算员工的月薪,以及一个 `get_bonus` 方法用于计算生日红包。然后我们定义了四个具体类,分别对应于四种不同类型的员工,它们都继承自 `Employee`,并实现了 `get_monthly_salary` 方法。最后我们创建了一个 `employees` 数组,保存了所有员工的信息,遍历这个数组,并依次调用每个员工的方法来显示工号、姓名、出生年月、月收入和红包。
阅读全文
相关推荐
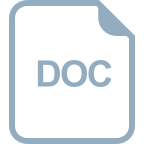
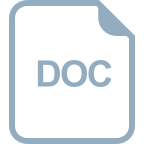










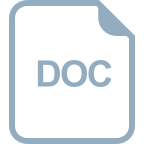
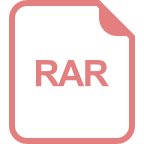
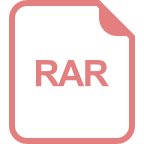
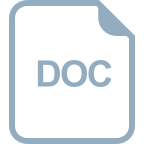
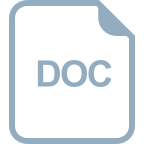
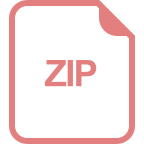