用Java写一个水仙花数
时间: 2024-04-11 07:07:45 浏览: 17
水仙花数是指一个三位数,其各位数字的立方和等于该数本身。例如:153=1^3+5^3+3^3。
下面是用 Java 来写一个水仙花数的示例代码:
```
public class NarcissisticNumber {
public static void main(String[] args) {
// 循环遍历100~999之间的数字
for (int i = 100; i <= 999; i++) {
// 分离出百位、十位、个位
int hundreds = i / 100;
int tens = (i / 10) % 10;
int units = i % 10;
// 判断是否为水仙花数
if (Math.pow(hundreds, 3) + Math.pow(tens, 3) + Math.pow(units, 3) == i) {
System.out.println(i + "是水仙花数");
}
}
}
}
```
在上面的代码中,我们使用一个循环遍历100~999之间的数字,然后使用整数除法和求余运算符来分离出百位、十位、个位。最后,使用 Math.pow() 函数来计算各位数字的立方和,并判断是否等于该数本身。
相关问题
用java写一个水仙花数
### 回答1:
你好! 下面是用 Java 写的一个程序来检测一个数是不是水仙花数:
```
public class NarcissisticNumber {
public static void main(String[] args) {
int num = 153; // 要检测的数字
int sum = 0; // 保存各位数字的立方和
int n = num; // 保存原数字
while (n > 0) {
int d = n % 10; // 取出个位数字
sum += d * d * d; // 累加立方和
n /= 10; // 去掉个位
}
if (sum == num) { // 如果立方和等于原数字
System.out.println(num + "是水仙花数");
} else {
System.out.println(num + "不是水仙花数");
}
}
}
```
希望这能帮到你!
### 回答2:
水仙花数是指一个三位数,其各位数字的立方和等于该数本身。
要用Java写一个水仙花数,可以按照以下步骤进行:
1. 创建一个新的Java类,命名为"DaffodilNumber"(或其他自定类名)。
2. 在主方法中添加代码,定义变量num,表示当前判断的数字。初始值为100。
3. 使用while循环,判断num是否小于1000。若满足条件,则执行循环体的代码。
4. 在循环体中,定义三个变量,分别为百位数digit1、十位数digit2、个位数digit3,通过对num进行取余和除法运算得到。
5. 使用Math.pow方法计算三个数字的立方和,赋值给变量result。
6. 判断如果result等于num,则输出num,即为一个水仙花数。
7. 在循环末尾,将num加1,以便判断下一个数字。
8. 运行程序,即可输出所有三位水仙花数。
下面是完整的Java代码示例:
```java
public class DaffodilNumber {
public static void main(String[] args) {
int num = 100;
while (num < 1000) {
int digit1 = num / 100; // 百位数
int digit2 = (num / 10) % 10; // 十位数
int digit3 = num % 10; // 个位数
double result = Math.pow(digit1, 3) + Math.pow(digit2, 3) + Math.pow(digit3, 3);
if (result == num) {
System.out.println(num);
}
num++;
}
}
}
```
运行程序后,即可输出所有三位的水仙花数:153、370、371、407。
### 回答3:
水仙花数是指一个三位数,其各个位数的立方和等于该数本身。例如,153是一个水仙花数,因为1³ + 5³ + 3³ = 153。
下面是用Java编写一个程序来找出所有的水仙花数:
```java
public class NarcissisticNumber {
public static void main(String[] args) {
System.out.println("水仙花数有:");
for (int i = 100; i < 1000; i++) {
int hundreds = i / 100; // 获取百位数
int tens = (i / 10) % 10; // 获取十位数
int units = i % 10; // 获取个位数
int cubeSum = (int) (Math.pow(hundreds, 3) + Math.pow(tens, 3) + Math.pow(units, 3));
if (cubeSum == i) {
System.out.println(i);
}
}
}
}
```
这段代码首先使用一个for循环遍历所有的三位数(从100到999)。然后,通过除以100、除以10并取余数的方式,分别获取百位数、十位数和个位数。然后,使用Math.pow()方法计算各个位数的立方和。如果立方和等于该三位数本身,就说明找到了一个水仙花数,并将其打印出来。
运行该程序,即可输出所有的水仙花数。
用Java写一个水仙花数算法
### 回答1:
水仙花数是指一个 3 位数,它的每个位上的数字的 3次幂之和等于它本身。例如:153=1^3+5^3+3^3。
以下是用 Java 写的水仙花数算法:
```
public class NarcissisticNumber {
public static void main(String[] args) {
for (int i = 100; i < 1000; i++) {
// 分离出百位、十位、个位
int a = i / 100;
int b = i / 10 % 10;
int c = i % 10;
if (i == a * a * a + b * b * b + c * c * c) {
// 打印水仙花数
System.out.println(i);
}
}
}
}
```
上面的代码会枚举 100 到 999 之间的所有数,并判断它们是否是水仙花数。如果是,就会打印出来。
### 回答2:
水仙花数是指一个n位数,它的每个位上的数字的n次幂之和等于它本身。例如,153是一个水仙花数,因为1^3 + 5^3 + 3^3 = 153。
下面是用Java写一个水仙花数算法的示例代码:
```java
public class NarcissisticNumber {
public static void main(String[] args) {
int n = 3; // 设置n的值,可以根据需求进行更改
System.out.println("水仙花数:");
findNarcissisticNumbers(n);
}
public static void findNarcissisticNumbers(int n) {
int start = (int) Math.pow(10, n - 1); // 起始值为10^(n-1)
int end = (int) Math.pow(10, n) - 1; // 结束值为10^n - 1
for (int i = start; i <= end; i++) {
if (isNarcissisticNumber(i)) {
System.out.println(i);
}
}
}
public static boolean isNarcissisticNumber(int number) {
int sum = 0;
int originalNumber = number;
int digitCount = String.valueOf(number).length();
while (number > 0) {
int digit = number % 10;
sum += Math.pow(digit, digitCount);
number /= 10;
}
return sum == originalNumber;
}
}
```
以上代码中,首先通过`findNarcissisticNumbers()`方法遍历区间范围内的整数,然后通过`isNarcissisticNumber()`方法判断每个整数是否为水仙花数。最后,将水仙花数打印出来。
可以通过在`main()`方法中更改变量`n`的值来设定需要查找水仙花数的位数。这样,在控制台就可以打印出相应位数的水仙花数。
### 回答3:
水仙花数,也称为阿姆斯特朗数,是指一个n位数(n≥3),它的每个数字的n次幂之和等于它本身。例如,153是一个水仙花数,因为1的3次方+5的3次方+3的3次方等于153。
要用Java写一个水仙花数算法,可以按照以下步骤进行:
1. 首先,确定要求水仙花数的范围,例如在1到1000之间寻找水仙花数。
2. 使用一个for循环来遍历这个范围内的每一个数字。
3. 在循环中,将当前数字存储在一个临时变量中。
4. 用一个while循环将临时变量除以10,直到临时变量变为0。在每一次循环中,取余数并将其与当前数字的每个位数的n次方的和相加。
5. 检查和是否等于当前数字。如果相等,则输出当前数字是水仙花数。
6. 继续循环,直到遍历完整个范围。
下面为示例代码:
```java
public class NarcissisticNumber {
public static void main(String[] args) {
int start = 1; // 范围起始数字
int end = 1000; // 范围结束数字
for (int i = start; i <= end; i++) {
int temp = i;
int sum = 0;
while (temp != 0) {
int remainder = temp % 10; // 取余数
sum += Math.pow(remainder, String.valueOf(i).length()); // 计算每个位数的n次方的和
temp /= 10; // 除以10,继续计算下一位数
}
if (sum == i) {
System.out.println(i + "是水仙花数");
}
}
}
}
```
这段代码会输出在1到1000之间的所有水仙花数。你可以根据需要调整起始数字和结束数字,运行代码验证结果。
阅读全文
相关推荐
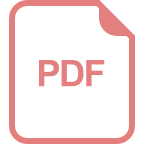
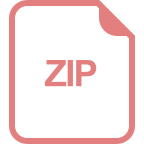
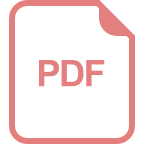





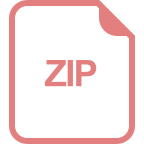
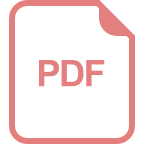
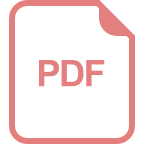
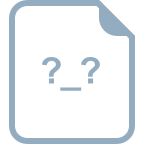
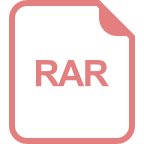
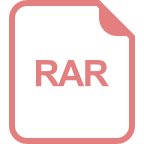
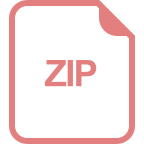