请使用geotools实现mbtiles的栅格瓦片写入操作
时间: 2024-03-21 11:40:27 浏览: 25
好的,以下是使用GeoTools实现MBTiles栅格瓦片写入的示例代码:
```java
import java.io.File;
import java.io.IOException;
import java.nio.file.Paths;
import java.util.HashMap;
import java.util.Map;
import org.geotools.data.DataStore;
import org.geotools.data.DataStoreFinder;
import org.geotools.data.DefaultTransaction;
import org.geotools.data.Transaction;
import org.geotools.data.mbtiles.MBTilesDataStoreFactory;
import org.geotools.data.mbtiles.MBTilesWriteAdapter;
import org.geotools.data.mbtiles.MBTilesWriteResult;
import org.geotools.factory.CommonFactoryFinder;
import org.geotools.factory.Hints;
import org.geotools.factory.ProjectFactoryFinder;
import org.geotools.factory.epsg.FactoryFinder;
import org.geotools.geometry.jts.JTSFactoryFinder;
import org.geotools.map.MapContent;
import org.geotools.referencing.CRS;
import org.geotools.renderer.GTRenderer;
import org.geotools.renderer.RenderListener;
import org.geotools.renderer.RendererUtilities;
import org.geotools.renderer.lite.StreamingRenderer;
import org.locationtech.jts.geom.Envelope;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import org.opengis.referencing.FactoryException;
import org.opengis.referencing.NoSuchAuthorityCodeException;
import org.opengis.referencing.crs.CoordinateReferenceSystem;
public class MBTilesRasterWriter {
public static void main(String[] args) throws Exception {
// 定义MBTiles文件路径
File mbtilesFile = new File("/path/to/mbtiles/file.mbtiles");
// 定义栅格瓦片数据源(例如GeoTIFF文件)
File rasterFile = new File("/path/to/raster/file.tif");
// 定义瓦片格式
String format = "png";
// 定义瓦片渲染范围
Envelope bbox = new Envelope(-180, 180, -90, 90);
// 定义瓦片数据范围(需要与栅格文件的范围一致)
Envelope dataExtent = new Envelope(-180, 180, -90, 90);
// 初始化MBTiles数据存储器
Map<String, Object> params = new HashMap<>();
params.put(MBTilesDataStoreFactory.PARAMETER_DBTYPE, "mbtiles");
params.put(MBTilesDataStoreFactory.PARAMETER_HOST, mbtilesFile);
DataStore store = DataStoreFinder.getDataStore(params);
// 初始化MBTiles写入适配器
MBTilesWriteAdapter writer = new MBTilesWriteAdapter(store.getDefaultSchema().getTypeNames()[0]);
// 构造瓦片数据类型
SimpleFeatureType tileType = writer.getTileType(dataExtent, bbox, format);
// 初始化渲染器
CoordinateReferenceSystem crs = CRS.decode("EPSG:4326");
GTRenderer renderer = new StreamingRenderer();
renderer.setContext(new MapContent());
renderer.getContext().setCRS(crs);
// 配置渲染参数
Hints hints = new Hints();
hints.put(Hints.FEATURE_DETACHED, Boolean.TRUE);
hints.put(Hints.DISPLAY_METHODS, new String[] { "STYLE" });
hints.put(Hints.PROJECT_FACTORY, ProjectFactoryFinder.getProjectFactory());
hints.put(Hints.JTS_COORDINATE_FACTORY, JTSFactoryFinder.getCoordinateFactory(null));
hints.put(Hints.JTS_GEOMETRY_FACTORY, JTSFactoryFinder.getGeometryFactory(null));
hints.put(Hints.RENDERING_ORDER, CommonFactoryFinder.getStyleFactory().getDefaultRenderingOrder());
hints.put(Hints.DEFAULT_COORDINATE_REFERENCE_SYSTEM, crs);
hints.put(Hints.COORDINATE_OPERATION_FACTORY, FactoryFinder.getCoordinateOperationFactory(hints));
hints.put(Hints.CRS_AUTHORITY_FACTORY, FactoryFinder.getCRSAuthorityFactory(hints));
hints.put(Hints.FEATURE_FACTORY, CommonFactoryFinder.getFeatureFactory(hints));
hints.put(Hints.STYLE_FACTORY, CommonFactoryFinder.getStyleFactory(hints));
hints.put(Hints.SIMPLE_FEATURE_FACTORY, CommonFactoryFinder.getFeatureFactory(hints));
hints.put(Hints.FILTER_FACTORY, CommonFactoryFinder.getFilterFactory());
hints.put(Hints.SEPARATED_THREAD_GROUP, true);
hints.put(Hints.MAXIMUM_VISIBLE_MEMORY, 20000000);
// 开始写入瓦片数据
Transaction tx = new DefaultTransaction();
try {
// 创建MBTiles数据表
writer.createTable(store, tileType, tx);
// 获取渲染后的瓦片图像,并写入MBTiles
MBTilesWriteResult result = writer.write(
new RenderListener[] { RendererUtilities.wrapSingleLayer(tileType) },
renderer,
rasterFile.toURI().toURL(),
dataExtent,
bbox,
format,
tx,
hints);
// 提交事务
tx.commit();
// 输出写入结果
System.out.println("Tiles written: " + result.getTileCount());
} catch (IOException e) {
// 回滚事务
tx.rollback();
throw e;
} finally {
tx.close();
store.dispose();
}
}
}
```
需要注意的是,以上代码中的`rasterFile`变量应该指向一个GeoTIFF格式的栅格文件。如果你的栅格文件不是GeoTIFF格式,可以使用GDAL等工具进行转换。此外,你也需要将代码中的`/path/to/mbtiles/file.mbtiles`和`/path/to/raster/file.tif`替换为实际的文件路径。
相关推荐
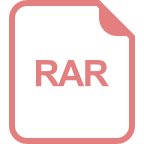
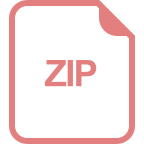
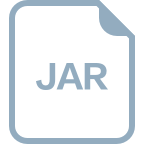














