使用vue写一个省市县三级联动
时间: 2023-12-05 16:05:06 浏览: 38
好的,以下是一个简单的省市县三级联动的Vue组件示例:
```html
<template>
<div>
<select v-model="selectedProvince" @change="provinceChange">
<option value="">请选择省份</option>
<option v-for="(province, index) in provinces" :key="index">{{ province.name }}</option>
</select>
<select v-model="selectedCity" @change="cityChange" :disabled="cities.length === 0">
<option value="">请选择城市</option>
<option v-for="(city, index) in cities" :key="index">{{ city.name }}</option>
</select>
<select v-model="selectedCounty" :disabled="counties.length === 0">
<option value="">请选择县区</option>
<option v-for="(county, index) in counties" :key="index">{{ county.name }}</option>
</select>
</div>
</template>
<script>
export default {
data() {
return {
provinces: [], // 所有省份列表
cities: [], // 当前选中省份的城市列表
counties: [], // 当前选中城市的县区列表
selectedProvince: "", // 当前选中的省份
selectedCity: "", // 当前选中的城市
selectedCounty: "", // 当前选中的县区
};
},
mounted() {
// 在组件挂载后获取所有省份列表
this.getProvinces();
},
methods: {
// 获取所有省份列表
getProvinces() {
this.provinces = [
{ name: "北京", code: "110000" },
{ name: "天津", code: "120000" },
{ name: "河北省", code: "130000" },
// 其他省份
];
},
// 根据当前选中的省份获取城市列表
async provinceChange() {
if (this.selectedProvince) {
// 发送异步请求获取当前省份的城市列表
const response = await fetch(`https://example.com/cities?province=${this.selectedProvince}`);
const data = await response.json();
this.cities = data;
this.counties = [];
this.selectedCity = "";
this.selectedCounty = "";
} else {
this.cities = [];
this.counties = [];
this.selectedCity = "";
this.selectedCounty = "";
}
},
// 根据当前选中的城市获取县区列表
async cityChange() {
if (this.selectedCity) {
// 发送异步请求获取当前城市的县区列表
const response = await fetch(`https://example.com/counties?city=${this.selectedCity}`);
const data = await response.json();
this.counties = data;
this.selectedCounty = "";
} else {
this.counties = [];
this.selectedCounty = "";
}
},
},
};
</script>
```
在上面的示例中,我们使用了三个 `select` 元素来展示省市县三级联动。当用户选择省份时,我们会通过异步请求获取该省份的城市列表并展示在第二个 `select` 元素中,同时清空第三个 `select` 元素的数据。当用户选择城市时,我们会通过异步请求获取该城市的县区列表并展示在第三个 `select` 元素中。最终,用户选择的省市县信息会保存在组件实例的 `selectedProvince`、`selectedCity` 和 `selectedCounty` 中。
相关推荐
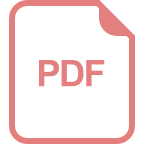
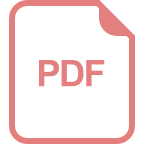














