1、设计一个学生信息管理系统,用于实现学生信息的录入、查询、排序、输出等功能。学生信息包括:学号(整型)、姓名(字符串)、性别(布尔型)、出生日期(结构体)、各科成绩(10门)、平均成绩等,用函数实现各功能 用面对对象
时间: 2023-10-20 15:24:31 浏览: 145
的编程思想,设计一个学生信息管理系统如下:
1. 定义一个学生类,包括私有成员变量:学号、姓名、性别、出生日期、各科成绩以及平均成绩;公有成员函数:构造函数、析构函数、输入信息函数、输出信息函数、计算平均成绩函数。
2. 定义一个学生信息管理类,包括私有成员变量:学生数组、学生数量;公有成员函数:构造函数、析构函数、添加学生函数、删除学生函数、查询学生函数、排序函数、输出所有学生信息函数。
具体实现如下:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
struct Date {
int year;
int month;
int day;
};
class Student {
private:
int id;
string name;
bool gender;
Date birth;
int score[10];
double average;
public:
Student():id(0),name(""),gender(true),birth({0,0,0}),average(0) {
for(int i=0; i<10; i++) {
score[i] = 0;
}
}
Student(int i, string n, bool g, Date b, int s[], double a):id(i),name(n),gender(g),birth(b),average(a) {
for(int i=0; i<10; i++) {
score[i] = s[i];
}
}
~Student() {}
void input() {
cout << "请输入学生信息:" << endl;
cout << "学号:";
cin >> id;
cout << "姓名:";
cin >> name;
cout << "性别(0代表女,1代表男):";
cin >> gender;
cout << "出生日期(格式为xxxx xx xx):";
cin >> birth.year >> birth.month >> birth.day;
cout << "各科成绩:";
for(int i=0; i<10; i++) {
cin >> score[i];
}
average = calcAverage();
}
void output() {
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << (gender?"男":"女") << endl;
cout << "出生日期:" << birth.year << "年" << birth.month << "月" << birth.day << "日" << endl;
cout << "各科成绩:";
for(int i=0; i<10; i++) {
cout << score[i] << " ";
}
cout << endl;
cout << "平均成绩:" << average << endl;
}
double calcAverage() {
int sum = 0;
for(int i=0; i<10; i++) {
sum += score[i];
}
return (double)sum / 10;
}
bool operator < (const Student &s) const {
return average < s.average;
}
bool operator == (const Student &s) const {
return id == s.id;
}
};
class StudentManager {
private:
Student *students;
int num;
public:
StudentManager():students(nullptr),num(0) {}
~StudentManager() {
if(students != nullptr) {
delete []students;
students = nullptr;
}
}
void addStudent() {
Student *newStudents = new Student[num+1];
for(int i=0; i<num; i++) {
newStudents[i] = students[i];
}
newStudents[num].input();
num++;
if(students != nullptr) {
delete []students;
}
students = newStudents;
}
void deleteStudent() {
int sid;
cout << "请输入要删除的学生的学号:" << endl;
cin >> sid;
for(int i=0; i<num; i++) {
if(students[i] == Student(sid,"",true,{0,0,0},{},0)) {
Student *newStudents = new Student[num-1];
for(int j=0; j<i; j++) {
newStudents[j] = students[j];
}
for(int j=i; j<num-1; j++) {
newStudents[j] = students[j+1];
}
num--;
if(students != nullptr) {
delete []students;
}
students = newStudents;
cout << "删除成功!" << endl;
return;
}
}
cout << "未找到该学生!" << endl;
}
void searchStudent() {
int sid;
cout << "请输入要查询的学生的学号:" << endl;
cin >> sid;
for(int i=0; i<num; i++) {
if(students[i] == Student(sid,"",true,{0,0,0},{},0)) {
students[i].output();
return;
}
}
cout << "未找到该学生!" << endl;
}
void sortStudents() {
sort(students, students+num);
}
void outputAllStudents() {
for(int i=0; i<num; i++) {
students[i].output();
}
}
};
int main() {
StudentManager sm;
int choice = 0;
while(true) {
cout << "请选择操作:" << endl;
cout << "1. 添加学生" << endl;
cout << "2. 删除学生" << endl;
cout << "3. 查询学生" << endl;
cout << "4. 排序学生" << endl;
cout << "5. 输出所有学生信息" << endl;
cout << "6. 退出" << endl;
cin >> choice;
switch(choice) {
case 1:
sm.addStudent();
break;
case 2:
sm.deleteStudent();
break;
case 3:
sm.searchStudent();
break;
case 4:
sm.sortStudents();
break;
case 5:
sm.outputAllStudents();
break;
case 6:
return 0;
default:
break;
}
}
return 0;
}
```
以上代码实现了一个简单的学生信息管理系统,可以通过用户输入选择不同的操作,包括添加学生、删除学生、查询学生、排序学生以及输出所有学生信息。通过面向对象的编程思想,将学生类和学生信息管理类进行了分离,使得代码结构更加清晰易懂。
阅读全文
相关推荐
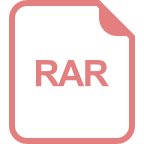
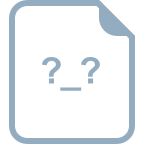
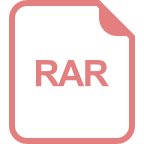
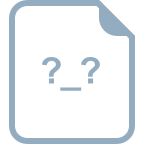









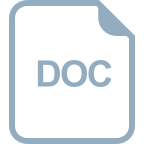
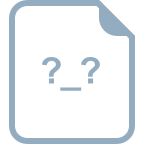
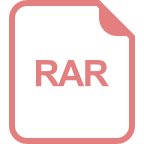