av_packet_rescale_ts
时间: 2023-09-04 12:11:02 浏览: 46
`av_packet_rescale_ts`是FFmpeg中的一个函数,用于重新计算音视频数据包(AVPacket)的时间戳(timestamp)和时基(time base)。它的原型如下:
```c
int av_packet_rescale_ts(AVPacket *pkt, AVRational tb_src, AVRational tb_dst);
```
其中,`pkt`是要处理的音视频数据包,`tb_src`是该数据包当前使用的时基,`tb_dst`是要转换到的目标时基。该函数会将`pkt`中的时间戳从`tb_src`时基下的值,转换为`tb_dst`时基下的值。
例如,如果一个视频数据包的时间戳是`1000`,当前使用的时基是`1/1000`,而我们需要将其转换为以`1/25`为时基的值,那么调用`av_packet_rescale_ts`的代码如下:
```c
AVRational src_timebase = {1, 1000}; // 当前使用的时基
AVRational dst_timebase = {1, 25}; // 要转换到的目标时基
av_packet_rescale_ts(pkt, src_timebase, dst_timebase);
```
调用该函数后,`pkt`中的时间戳会被转换为`40`,以`1/25`为时基的值。
注意,该函数不会修改数据包中的时间基准(base),因此在使用`av_packet_rescale_ts`转换时间戳时,需要保证数据包中的时间基准正确无误。
相关问题
解释一下 ffmpeg接口 av_packet_rescale_ts
ffmpeg接口中的av_packet_rescale_ts函数可以将packet的时间戳重新计算为相对于新的时间基准的时间戳。在音视频处理中,我们通常会遇到需要更改时间基准的情况,比如切换视频分辨率、改变播放速度等。此时,就需要使用av_packet_rescale_ts函数来重新计算包的时间戳,以保证实际的播放时间与音视频的时间戳匹配。
av_interleaved_write_frame使用方法
`av_interleaved_write_frame`是FFmpeg库中用于向输出文件写入音频/视频帧的函数。它的使用方法与以下步骤相似:
1. 创建一个AVPacket数据包,将待写入的音频/视频数据填充到该数据包中。可以使用函数`av_packet_alloc()`来创建一个AVPacket数据包,使用`av_packet_from_data()`函数将音频/视频数据填充到该数据包中。
2. 设置AVPacket数据包的时间戳和持续时间。这可以通过调用`av_packet_rescale_ts()`函数来完成,该函数将时间戳和持续时间从输入格式的时间基转换为输出格式的时间基。
3. 将AVPacket数据包写入输出文件。这可以通过调用`av_interleaved_write_frame()`函数来完成,该函数将AVPacket数据包写入输出文件。
以下是使用`av_interleaved_write_frame()`函数将音频/视频帧写入输出文件的示例代码:
```c
AVFormatContext *output_format_context;
AVStream *output_stream;
AVCodecContext *output_codec_context;
/* 创建AVPacket数据包 */
AVPacket *packet = av_packet_alloc();
if (!packet) {
// 错误处理
}
/* 将音频/视频数据填充到AVPacket数据包中 */
// ...
/* 设置AVPacket数据包的时间戳和持续时间 */
packet->pts = av_rescale_q_rnd(packet->pts, input_stream->time_base, output_stream->time_base, AV_ROUND_NEAR_INF|AV_ROUND_PASS_MINMAX);
packet->dts = av_rescale_q_rnd(packet->dts, input_stream->time_base, output_stream->time_base, AV_ROUND_NEAR_INF|AV_ROUND_PASS_MINMAX);
packet->duration = av_rescale_q(packet->duration, input_stream->time_base, output_stream->time_base);
packet->stream_index = output_stream->index;
/* 将AVPacket数据包写入输出文件 */
int ret = av_interleaved_write_frame(output_format_context, packet);
if (ret < 0) {
// 错误处理
}
/* 释放AVPacket数据包 */
av_packet_unref(packet);
```
需要注意的是,使用`av_interleaved_write_frame()`函数写入音频/视频帧时,必须保证音频/视频帧已经被编码为输出格式所支持的编码格式,并且输出格式的AVCodecContext已经被正确地设置。否则,写入的音频/视频帧可能无法被输出文件所支持,并且可能会导致写入失败。
相关推荐
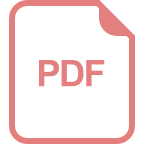













