1067 - Invalid default value for 'OPERATE_TIME'
时间: 2023-08-06 09:05:43 浏览: 58
这个错误通常发生在 MySQL 数据库中创建表格时,因为 `OPERATE_TIME` 的默认值不符合 MySQL 的要求。在 MySQL 中,时间戳列(如 `OPERATE_TIME`)的默认值必须是 `CURRENT_TIMESTAMP` 或者 `DEFAULT CURRENT_TIMESTAMP`。
要解决这个问题,你需要修改 `OPERATE_TIME` 的默认值为 `CURRENT_TIMESTAMP`。下面是一个示例代码:
```
CREATE TABLE example_table (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
operate_time TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (id)
);
```
在上面的示例中,`operate_time` 列的默认值被设置为 `CURRENT_TIMESTAMP`,这样就避免了出现 `Invalid default value for 'OPERATE_TIME'` 的错误。
相关问题
> 1067 - Invalid default value for 'begin_time'
这个错误通常是因为 MySQL 版本不兼容导致的。在 MySQL 5.7.5 之前,datetime 类型的字段默认值不能为 NULL,必须指定一个默认值。可以尝试将默认值改为当前时间戳,例如:
```
begin_time TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP
```
如果你使用的是 MySQL 5.7.5 或更高版本,可以将 sql_mode 设置为 NO_ZERO_IN_DATE,NO_ZERO_DATE,例如:
```
SET sql_mode='NO_ZERO_IN_DATE,NO_ZERO_DATE';
```
1067 - Invalid default value for 'CREATE_PRG_ID'
根据提供的引用内容,我们可以看出这是关于MySql的错误。错误信息是“1067 - Invalid default value for 'CREATE_PRG_ID'”,这意味着在创建表时,'CREATE_PRG_ID'列的默认值无效。这通常是由于在创建表时使用了不受支持的数据类型或格式导致的。
要解决此问题,您可以尝试以下几个步骤:
1. 检查表的创建语句,确保所有列都具有正确的数据类型和格式。
2. 检查是否使用了不受支持的数据类型或格式。
3. 如果使用了日期或时间戳,请确保它们具有正确的格式。
4. 如果使用了默认值,请确保它们具有正确的格式和值。
以下是一个示例表创建语句,其中包含一个名为'CREATE_PRG_ID'的列:
```sql
CREATE TABLE example_table (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
CREATE_PRG_ID TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (id)
);
```
如果您仍然无法解决问题,请提供更多关于表结构和错误消息的详细信息,以便我们更好地帮助您解决问题。
相关推荐
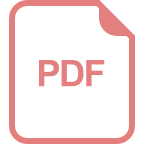
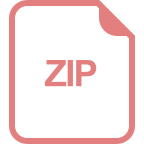
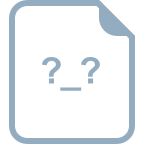












