Code Review for Insufficient MATLAB Input Parameters: Enhancing Code Quality and Team Collaboration
发布时间: 2024-09-14 14:42:36 阅读量: 9 订阅数: 16 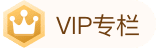
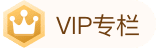
# Overview of Insufficient Input Parameters in MATLAB: Improving Code Quality and Team Collaboration
## 1. Definition and Impact of Insufficient Input Parameters
Insufficient input parameters refer to the absence of necessary parameters when a function or script is called. This can lead to code execution failure or unexpected results, thereby reducing code quality and reliability.
## 2. Theoretical Foundations: Best Practices for MATLAB Input Parameters
### Types and Validation of Input Parameters
Input parameters in MATLAB functions can be of various types, including:
- **Numeric types:** integers (int), floating-point numbers (double), complex numbers (complex)
- **String types:** character arrays (char)
- **Struct types:** collections containing different types of data
- **Cell array types:** collections containing different types of data, where each element can be any type
To ensure the validity of input parameters, MATLAB provides various validation mechanisms:
- **Type checking:** Verifies if the input parameters match the expected types.
- **Range checking:** Verifies if the input parameters are within a specified range.
- **Non-empty checking:** Verifies if the input parameters are not empty.
- **Regular expression checking:** Verifies if the input parameters match a specified regular expression pattern.
### Default Values and Optional Parameters
MATLAB allows for the specification of default values for input parameters, which can prevent insufficient input parameters. Default values are specified in the function definition, and if a parameter is not provided during function calls, the default value is used.
Optional parameters allow functions to run without providing certain parameters. Optional parameters are specified in the function definition and collected using the `varargin` or `varargout` variables.
#### Using Default Values to Avoid Insufficient Input Parameters
```
function myFunction(x, y, z)
% Set default values
if nargin < 2
y = 0;
end
if nargin < 3
z = 1;
end
% Function logic
end
```
In the example above, if the `y` or `z` parameters are not provided during the function call, the default values of 0 and 1 are used, respectively.
#### Defining Optional Parameters to Enhance Flexibility
```
function myFunction(x, varargin)
% Parse optional parameters
p = inputParser;
addParameter(p, 'option1', 'default1');
addParameter(p, 'option2', 'default2');
parse(p, varargin{:});
% Function logic
end
```
In the example above, the function accepts a required parameter `x` and an arbitrary number of optional parameters. The optional parameters are parsed using `inputParser` and stored in the `p.Results` structure.
# 3. Practical Guide: Input Parameter Checks in Code Review
### Manual Code Review
#### Checking Function Definitions and Calls
The first step in manual code review is to check the function definitions and calls. Ensure that all required input parameters are specified in the function definition and that the corresponding values are provided during function calls. For example:
```matlab
function myFunction(x, y, z)
% Function body
end
myFunction(1, 2) % Missing input argument 'z'
```
In the example above, `myFunction` defines three input parameters, but only two parameters are provided in the function call, resulting in insufficient input parameters.
#### Using MATLAB Linter Tools
MATLAB linter tools (e.g., MLint) can help identify potential issues with insufficient input parameters. These tools inspect the code and look for unused variables, duplicated code, and other potential issues, including insufficient input parameters.
### Automated Code Review
#### Using Static Code Analysis Tools
Static code analysis tools (e.g., Coverity, SonarQube) can automatically scan the code and identify insufficient input parameters and other defects. These tools use advanced algorithms to analyze the code structure and data flow to detect potential problems.
#### Integrating with Continuous Integration/Continuous Delivery (CI/CD) Pipelines
Integrating the review of insufficient input parameters into CI/CD pipelines can achieve automated and continuous code quality checks. CI/CD pipelines can automatically run static code analysis tools after every code change
0
0
相关推荐
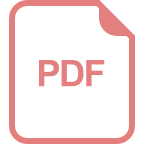
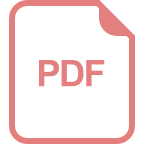






