Debugging Techniques for Insufficient MATLAB Input Parameters: Quickly Locate and Solve Problems
发布时间: 2024-09-14 14:39:31 阅读量: 37 订阅数: 31 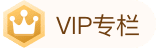
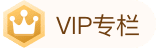
# 1. Overview of MATLAB Insufficient Input Parameters**
When calling MATLAB functions, insufficient input parameters can lead to the function not executing properly. This error can be difficult to locate, as MATLAB does not provide explicit error messages. This guide will introduce techniques for debugging MATLAB insufficient input parameters, helping you quickly locate and resolve the issue.
Debugging insufficient input parameters involves understanding the MATLAB function calling mechanism and input parameter type checking. By using the `nargin` function to check the number of input parameters, the `error` function to throw error messages, and `varargin` and `varargout` to handle variable arguments, you can effectively debug insufficient input parameter issues.
# 2. Theoretical Basis for Debugging Insufficient Input Parameters
### 2.1 MATLAB Function Calling Mechanism
The MATLAB function calling mechanism follows these steps:
1. **Parse the function definition:** MATLAB parses the function definition to determine the function name, input parameter list, and output parameter list.
2. **Pass input parameters:** When the function is called, the actual input parameters are passed to the function's input parameter list.
3. **Check input parameter types:** MATLAB checks if the actual input parameter types match the input parameter types defined in the function.
4. **Execute the function body:** If the input parameter types match, MATLAB executes the function body.
5. **Return output parameters:** Once the function execution is complete, the output parameters are returned to the calling function.
### 2.2 Input Parameter Type Checking
MATLAB uses the following rules to check input parameter types:
| Data Type | Matching Rule |
|---|---|
| Numeric | Any numeric type (int, float, complex) |
| String | Any string type (char, string) |
| Cell Array | Any cell array type (cell) |
| Structure | Any structure type (struct) |
| Object | Any object type (class) |
If the actual input parameter types do not match the input parameter types defined in the function, MATLAB will throw an error.
# 3. Practical Methods for Debugging Insufficient Input Parameters**
### 3.1 Use the `nargin` Function to Check the Number of Input Parameters
The `nargin` function provided by MATLAB returns the number of parameters passed to the current function. By checking the value of `nargin`, we can determine if the number of passed parameters is insufficient.
```matlab
function myFunction(a, b, c)
if nargin < 3
error('Insufficient input arguments.');
end
% Function logic
end
```
**Code Logic Analysis:**
* If the number of passed parameters is less than 3, the value of `nargin` will be less than 3, and the function will throw an error message.
* If the number of passed parameters meets the requirement, the function will continue to execute its logic.
### 3.2 Use the `error` Function to Throw Error Messages
The `error` function can be used to throw custom error messages. When insufficient input parameters are detected, we can use the `error` function to throw a clear error message, helping users quickly locate the problem.
```matlab
function myFunction(a, b
```
0
0
相关推荐








