Error Handling for Insufficient Input Parameters in MATLAB: Gracefully Managing Exceptional Situations
发布时间: 2024-09-14 14:40:29 阅读量: 40 订阅数: 26 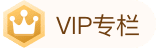
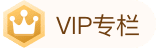
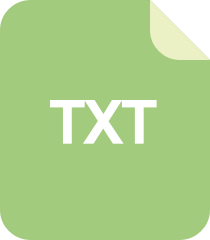
解决Installation error: INSTALL_FAILED_INSUFFICIENT_STORAGE

# Handling Insufficient Input Parameters in MATLAB: Gracefully Managing Exceptional Cases
## 1. Overview of Insufficient Input Parameters in MATLAB
When calling MATLAB functions, an error is triggered if the number of provided input parameters is less than required. This error typically occurs because the function definition specifies more parameters than those actually provided.
An error due to insufficient input parameters can interrupt the execution of a function and cause the program to crash. Therefore, it is crucial to properly manage cases of insufficient input parameters when writing MATLAB functions.
## 2. Strategies for Handling Insufficient Input Parameters**
**2.1 Using Default Values**
A simple error-handling strategy when functions lack certain input parameters is to use default values. These are specified in the function definition and are used when actual parameters are not provided. The advantage of using default values is that it allows the function to remain usable even if not all necessary inputs are present.
```
% Define the function
function myFunction(x, y, z)
% Set default values
if nargin < 2
y = 0;
end
if nargin < 3
z = 0;
end
% Calculate the result
result = x + y + z;
end
```
**Code Logic Analysis:**
* The `nargin` function returns the number of input parameters passed to the function.
* If `nargin` is less than 2, `y` is set to the default value of 0.
* If `nargin` is less than 3, `z` is set to the default value of 0.
* The function uses the `result` variable to store the sum of `x`, `y`, and `z`.
**2.2 Using Input Prompts**
Another error-handling strategy is to use input prompts. Input prompts prompt the user to provide missing input parameters. The user must enter the parameters to continue executing the function. The advantage of using input prompts is that it ensures the function has all the required inputs.
```
% Define the function
function myFunction(x, y, z)
% Check the number of input parameters
if nargin < 3
% Prompt the user to enter z
z = input('Please enter z: ');
end
% Calculate the result
result = x + y + z;
end
```
**Code Logic Analysis:**
* If `nargin` is less than 3, the user is prompted to enter `z`.
* The user must input `z` to continue executing the function.
* The function uses the `result` variable to store the sum of `x`, `y`, and `z`.
**2.3 Using Variable Argument Lists**
Variable argument lists allow functions to accept an arbitrary number of input parameters. Variable argument lists are represented by the `varargin` keyword. The advantage of using variable argument lists is that it makes the function flexible in handling different numbers of inputs.
```
% Define the function
function myFunction(varargin)
% Check the number of input parameters
if nargin == 0
error('At least one input parameter must be provided.');
end
% Extract the first parameter
x = varargin{1};
% Calculate the result
result = x;
for i = 2:nargi
```
0
0
相关推荐
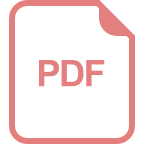
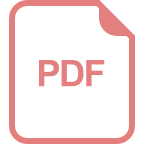
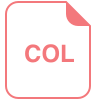
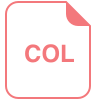
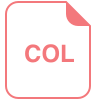
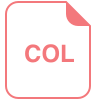
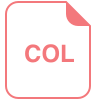
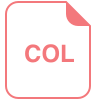