Books Recommended for Inadequate Input Parameters in MATLAB: A Deep Dive into Theory and Practice
发布时间: 2024-09-14 14:49:31 阅读量: 23 订阅数: 26 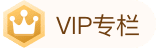
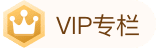
# 1. Overview of Insufficient Input Parameters in MATLAB
In MATLAB, functions and scripts typically require input parameters to run properly. When these parameters are insufficient, MATLAB will report errors or return unexpected results. Insufficient input parameters are common in MATLAB programming and can cause program crashes or inaccurate results if not handled correctly. Therefore, understanding how to handle insufficient input parameters in MATLAB is crucial.
This guide will delve into the theoretical and practical applications of dealing with insufficient input parameters in MATLAB. We will cover the types and roles of input parameters, methods for handling insufficient input parameters, and practical applications in common tasks such as file processing, image processing, and data analysis. By understanding these concepts, you can effectively manage insufficient input parameters in MATLAB and write robust, reliable programs.
# 2. Theoretical Basis of Insufficient Input Parameters in MATLAB
### 2.1 Types and Roles of Input Parameters
Input parameters in MATLAB functions can be classified into the following types:
**2.1.1 Scalar Input**
Scalar input is the most basic type, representing a single value such as a number, string, or boolean. Scalar input is usually used to specify specific settings or options for a function.
```
% Calculate the area of a circle
function area = circle_area(radius)
% Input parameters:
% radius: the radius of the circle
% Calculate the area
area = pi * radius^2;
end
```
**2.1.2 Matrix Input**
Matrix input represents a two-dimensional array of numbers. Matrix input is often used to represent structured data such as data or images.
```
% Convert an image to grayscale
function grayscale_image = rgb2gray(rgb_image)
% Input parameters:
% rgb_image: an RGB image
% Extract the luminance channel
grayscale_image = rgb_image(:,:,1) * 0.299 + rgb_image(:,:,2) * 0.587 + rgb_image(:,:,3) * 0.114;
end
```
**2.1.3 Structured Input**
Structured input represents a composite data type containing multiple fields. Structured input is typically used to organize and store related data.
```
% Create a structure containing student information
function student = create_student_struct(name, age, major)
% Input parameters:
% name: the student's name
% age: the student's age
% major: the student's major
% Create the structure
student = struct('name', name, 'age', age, 'major', major);
end
```
### 2.2 Methods for Handling Insufficient Input Parameters
When there are insufficient input parameters for a function, MATLAB provides several methods for handling them:
**2.2.1 Default Value Settings**
Default values can be set for input parameters to handle insufficient input parameters. These default values will be used automatically during function calls unless explicit values are provided.
```
% Calculate the area of a circle, default radius is 1
function area = circle_area(radius)
% Input parameters:
% radius: the radius of the circle (optional, default value is 1)
% Set the default value
if nargin < 1
radius = 1;
end
% Calculate the area
area = pi * radius^2;
end
```
**2.2.2 Optional Parameters**
Optional parameters allow users to provide or omit specific input parameters during function calls. Optional parameters typically use the `varargin` variable to represent them.
```
% Calculate the area and circumference of a circle, the circumference parameter is optional
function [area, circumference] = circle_properties(radius, circumference_flag)
% Input parameters:
% radius: the radius of the circle
% circumference_flag: whether to calculate the circumference (optional, default value is false)
% Set the default value
if nargin < 2
circumference_flag = false;
end
% Calculate the area
area = pi * radius^2;
% Calculate the circumference if needed
if circumference_flag
circumference = 2 * pi * radius;
end
end
```
**2.2.3 Error Handling**
When there are insufficient input parameters and they cannot be handled by default values or optional parameters, error handling can be used to address the issue. Error handling allows the function to throw exceptions when errors are detected.
```
% Calculate the area of a circle, throw an error if the radius is negative
function area = circle_area(radius)
% Input parameters:
% radius: the radius of the circle
% Validate input parameters
if radius < 0
error('Radius cannot be negative');
end
% Calculate the area
area = pi * radius^2;
end
```
# 3. Practical Applications of Insufficient Input Parameters in MATLAB
### 3.1 Insufficient Input Parameters in File Processing
File processing is a common task in MATLAB, involving reading, writing, and manipulating files. Insufficient input parameters in file processing can lead to various problems, such as the file not being able to open, data loss, or program crashes.
#### 3.1.1 File Reading
File reading operations
0
0
相关推荐
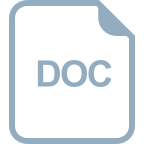
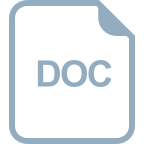
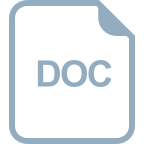
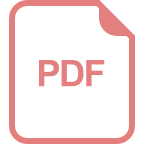
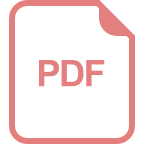
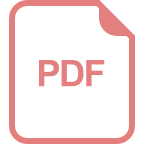
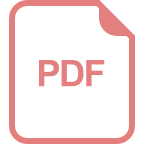
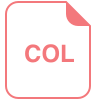
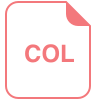