Relational Operators in MATLAB: The Basics of Conditional Comparison (with 50 Practical Examples)
发布时间: 2024-09-13 18:12:47 阅读量: 26 订阅数: 27 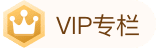
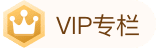
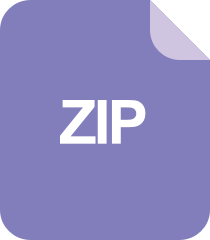
Relational Join: 两个矩阵的关系连接-matlab开发
# Relational Operators in MATLAB: The Basics of Conditional Comparison (With 50 Real-World Examples)
## 1. Relational Operators in MATLAB
Relational operators in MATLAB are used to compare two values or expressions. They return a boolean value (true or false) ***monly used relational operators in MATLAB include:
- Equal to (==): Compares if two values are equal.
- Not equal to (~=): Compares if two values are not equal.
- Greater than (>): Compares if the left value is greater than the right value.
- Less than (<): Compares if the left value is less than the right value.
- Greater than or equal to (>=): Compares if the left value is greater than or equal to the right value.
- Less than or equal to (<=): Compares if the left value is less than or equal to the right value.
## 2. Applying Relational Operators: Conditional Judgments
Relational operators can be used not only for numerical comparison but also for conditional judgments. Conditional judgments are an essential means of controlling the execution flow of programs, and this chapter will detail the application of relational operators in conditional judgments.
### 2.1 Basic Syntax of Conditional Judgments
The basic syntax of conditional judgments is as follows:
```matlab
if condition expression
statement block 1
elseif condition expression
statement block 2
else
statement block n
end
```
Where:
- `if`: The starting marker for a conditional statement.
- `condition expression`: An expression used to determine if the condition is true, executing the subsequent statement block if the result is true (non-zero).
- `statement block`: The code block to be executed when the condition is met.
- `elseif`: Optional, used to add multiple conditional judgments, executing the next condition when the previous one is not met.
- `else`: Optional, the statement block to be executed when none of the conditions are met.
### 2.2 Using Logi***
***mon logical operators include:
| Operator | Meaning |
|---|---|
| `&&` | AND operation, the result is true only when both conditions are met |
| `||` | OR operation, the result is true if at least one of the conditions is met |
| `~` | NOT operation, inverts the condition, the result is true when the condition is false, and vice versa |
For example:
```matlab
if x > 0 && y < 10
% Execute statement block
end
```
This conditional statement indicates that when `x` is greater than 0 and `y` is less than 10, the statement block will be executed.
### 2.3 Nested Conditional Judgments
Conditional judgments can be nested to create more complex logic. The syntax for nested conditional judgments is:
```matlab
if condition expression 1
statement block 1
if condition expression 2
statement block 2
else
statement block 3
end
else
statement block 4
end
```
For example:
```matlab
if x > 0
if y < 10
% Execute statement block 1
else
% Execute statement block 2
end
else
% Execute statement block 3
end
```
This conditional judgment indicates that when `x` is greater than 0, it checks whether `y` is less than 10. If it is, statement block 1 is executed; otherwise, statement block 2 is executed. If `x` is not greater than 0, statement block 3 is executed.
### 2.4 Common Errors in Conditional Judgments
Some common mistakes when using conditional judgments include:
***Incorrect condition expression:** The condition expression must be a logical expression, resulting in true or false.
***Improper use of logical operators:** It is important to pay attention to the precedence and associativity of logical operators to avoid logical errors.
***Excessive nested condition judgments:** Excessive nested conditions can make the code difficult to read and maintain, and should be avoided as much as possible.
***Inadequate condition judgments:** Condition judgments should consider all possible scenarios to avoid omissions or misjudgments.
By mastering the application of relational operators, complex conditional judgments can be implemented to control the program's execution flow, thus writing more robust and flexible code.
## 3.1 Numerical Comparison and Judgment
#### 3.1.***
***mon numerical magnitude comparison operators include:
- `>`: Greater than
- `<`: Less than
- `>=`: Greater than or equal to
- `<=`: Less than or equal to
**Example:**
```matlab
% Comparing two numbers
a = 5;
b = 3;
result = a > b; % The result is true
```
**Line-by-line code logic interpretation:**
- Line 1: Define variable `a` and assign it a value of 5.
- Line 2: Define variable `b` and assign it a value of 3.
- Line 3: Use the `>` operator to compare the magnitude of `a` and `b`, and store the result in the variable `result`. Since `a` is greater than `b`
0
0
相关推荐







