Conditional Plotting in MATLAB: Visualizing Data Based on Conditions (with 15 Application Scenarios)
发布时间: 2024-09-13 18:20:24 阅读量: 24 订阅数: 27 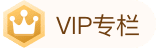
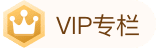
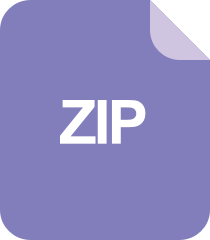
Conditional Nonparametric Kernel Density:Condtional Kernel Density Estimate with normal kernel and LSCV带宽选择-matlab开发
# 1. Overview of MATLAB Conditional Plotting**
Conditional plotting is a powerful technique in MATLAB that allows for visualizing data based on specific conditions. By utilizing conditional statements and functions, you can create complex plots that dynamically change according to data values. Conditional plotting is widely used in various applications such as data exploration, image processing, and signal analysis.
MATLAB provides a range of conditional plotting functions, including if-else statements, switch-case statements, logical() functions, and find() functions. These functions enable you to set conditions based on data values and draw different graphical elements accordingly. For instance, you can use if-else statements to plot data points that meet specific conditions, or use switch-case statements to plot data points of different categories.
# 2. Basic Syntax and Functions of Conditional Plotting
### 2.1 Conditional expressions in plotting
In conditional plotting, conditional expressions are used to define the conditions for drawing or not drawing a graph. Conditional expressions can be any valid MATLAB logical expression, including:
- Comparison operators (==, ~=, <, >, <=, >=)
- Logical operators (&, |, ~)
- Boolean values (true, false)
- Relational operators (isnumeric, isempty)
### 2.2 Common conditional plotting functions
MATLAB offers several conditional plotting functions to draw different graphical elements based on conditions. Here are some of the most commonly used functions:
#### 2.2.1 if-else statements
```matlab
if condition
% Plot graph 1
else
% Plot graph 2
end
```
**Parameter Description:**
* `condition`: A conditional expression; if true, the first block of code is executed; otherwise, the second block of code is executed.
**Code Logic:**
The if-else statement divides the code into two branches based on the conditional expression. If the condition is true, the first block of code is executed, plotting graph 1; if not, the second block is executed, plotting graph 2.
#### 2.2.2 switch-case statements
```matlab
switch variable
case value1
% Plot graph 1
case value2
% Plot graph 2
otherwise
% Plot graph 3
end
```
**Parameter Description:**
* `variable`: The variable to be evaluated.
* `value1`, `value2`: Values compared with `variable`.
* `otherwise`: The code block to be executed if `variable` does not match any specified value.
**Code Logic:**
The switch-case statement divides the code into multiple branches based on the value of `variable`. If the value of `variable` equals `value1`, the first block of code is executed, plotting graph 1; if it equals `value2`, the second block is executed, plotting graph 2; otherwise, the `otherwise` block is executed, plotting graph 3.
#### 2.2.3 logical() function
```matlab
logical_condition = logical(condition);
if logical_condition
% Plot graph
end
```
**Parameter Description:**
* `condition`: The conditional expression to be converted into a logical value.
**Code Logic:**
The logical() function converts the conditional expression into a boolean value. If the conditional expression is true, `logical_condition` is true; otherwise, it is false. The if statement then decides whether to plot the graph based on the value of `logical_condition`.
#### 2.2.4 find() function
```matlab
indices = find(condition);
% Plot the graph using indices as an index
```
**Parameter Description:**
* `condition`: The conditional expression to be evaluated.
**Code Logic:**
The find() function returns the indices of elements that satisfy the conditional expression. These indices can then be used to plot a graph, for example, using the plot() function to plot a scatter plot of specific data points.
# 3.1 Multi-Condition Plotting
In MATLAB, multi-condition plotting can be created using multiple conditional expressions to visualize data according to multiple conditions, thus providing deeper insights.
#### Logical operators
MATLAB provides a set of logical operators for combining multiple conditional expressions. These operators include:
* `&&`: Logical AND operator. The result is true if both conditions are true.
* `||`: Logical OR operator. The result is true if at least one condition is true.
* `~`: Logical NOT operator. It inverts the condition.
#### Multi-condition plotting syntax
The syntax for multi-condition plotting using multiple conditional expressions is as follows:
```matlab
if condition1 && condition2
% Execute code block 1
elseif condition3
% Execute code block 2
else
% Execute code block 3
end
```
Where:
* `condition1`, `condition2`, `condition3` are conditional expressions.
* `%` denotes a comment.
* `end` ends the conditional statement.
#### Example
The following example demonstrates how to use multi-condition plotting to visualize data that meets multiple conditions:
```matlab
% Generate data
x = 1:100;
y = randn(1, 100);
% Create a multi-condition plot
figure;
plot(x, y, 'b-');
hold on;
% Plot data points that meet the condition y > 0
plot(x(y >
```
0
0
相关推荐







