Advanced Guide to MATLAB if Statements: Handling Complex Conditions and Nested Statements (10 Practical Examples)
发布时间: 2024-09-13 18:09:31 阅读量: 21 订阅数: 23 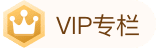
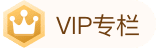
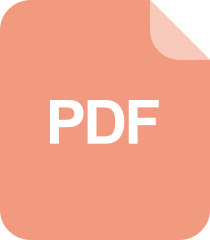
JavaScript in 10 Minutes
# 1. Advanced MATLAB if Statement Guide: Handling Complex Conditions and Nested Statements (10 Real-world Examples)
MATLAB's `if` statement is a control flow statement that allows code execution based on a condition. Its fundamental syntax is as follows:
```matlab
if condition
statements
end
```
Where:
- `condition` is a Boolean expression that evaluates to `true` or `false`.
- `statements` are the code blocks executed when `condition` is `true`.
# 2. Dealing with Complex Conditions
When handling complex conditions, MATLAB offers a variety of tools, including logical operators, nested if statements, and switch-case statements.
### 2.1 Logical Operators and Precedence
Logical operators are used to combine multiple conditions, including:
- `&&` (AND): The result is true if all conditions are true.
- `||` (OR): The result is true if any condition is true.
- `~` (NOT): Reverses true to false and false to true.
The precedence of logical operators is as follows (from highest to lowest):
1. `~`
2. `&&`
3. `||`
For example, the following code checks if a number is even:
```matlab
x = 10;
if mod(x, 2) == 0 && x > 0
disp('x is even')
end
```
### 2.2 Nested if Statements
Nested if statements allow creating other if statements within an if statement. This enables the creation of complex branching logic.
For example, the following code checks if a number is a positive even number:
```matlab
x = 10;
if x > 0
if mod(x, 2) == 0
disp('x is a positive even number')
end
end
```
### 2.3 Using switch-case Statements
The switch-case statement is used to execute different operations based on the value of a variable. It is similar to if-else statements but more concise, especially when dealing with multiple conditions.
For example, the following code uses a switch-case statement to print grades based on a letter grade:
```matlab
grade = 'A';
switch grade
case 'A'
disp('Excellent')
case 'B'
disp('Good')
case 'C'
disp('Average')
case 'D'
disp('Pass')
case 'F'
disp('Fail')
otherwise
disp('Invalid grade')
end
```
| Grade | Evaluation |
|---|---|
| A | Excellent |
| B | Good |
| C | Average |
| D | Pass |
| F | Fail |
# 3. Practical Applications of Nested Statements
### 3.1 Identifying Odd and Even Numbers
Identifying odd and even numbers is a common application of if statements. The following code demonstrates how to use if statements for this purpose:
```matlab
% Get user input for a number
number = input('Enter a number: ');
% Check if the number is odd
if mod(number, 2) == 1
% If the number is odd, print "Odd"
disp('The number is odd.');
else
% If the number is even, print "Even"
disp('The number is even.');
end
```
**Logic Analysis of the Code:**
* `mod(number, 2)` computes the remainder when number is divided by 2. If the remainder is 1, then number is odd.
* The `if` statement checks if the remainder is 1. If so, it executes `disp('The number is odd.')`.
* The `else` statement handles the case where the remainder is not 1, meaning the number is even, and executes `disp('The number is even.')`.
### 3.2 Calculating Factorial
Factorial calculation is another common application of if statements. The following code demonstrates how to use if statements for this purpose:
```matlab
% Get user input
```
0
0
相关推荐
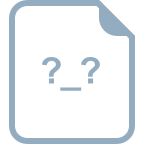
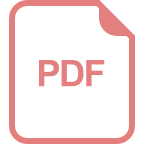
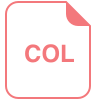
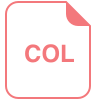
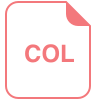
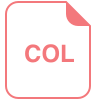
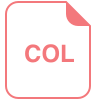
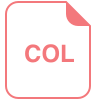
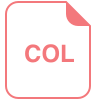