Unveiling the Secrets of MATLAB if Statements: Mastering the Art of Conditional Judgment
发布时间: 2024-09-13 18:08:08 阅读量: 22 订阅数: 23 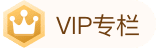
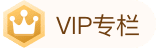
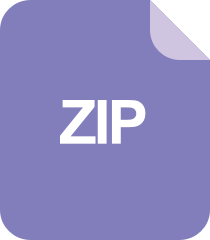
zip4j.jar包下载,版本为 2.11.5
# Unveiling the Secrets of MATLAB if Statements: Mastering the Art of Conditional Judgement
MATLAB if statements are powerful tools for conditional judgement, used to control program flow and execute different blocks of code based on specific conditions. The basic syntax of an if statement is as follows:
```
if condition_expression
% Code block to be executed if the condition is true
end
```
The condition expression is a Boolean expression that can be evaluated as true or false. If the condition expression is true, the code block within the if statement is executed; otherwise, the code block is skipped.
# 2. Conditional Judgement in if Statements
### 2.1 Basic Conditional Operators
The basic conditional operators in MATLAB are used to compare two values and return a Boolean value (true or false). These operators include:
- Equal to (==): Compares if two values are equal.
- Not equal to (~=`): Compares if two values are not equal.
- Greater than (`>`): Compares if the first value is greater than the second value.
- Less than (`<`): Compares if the first value is less than the second value.
- Greater than or equal to (`>=`): Compares if the first value is greater than or equal to the second value.
- Less than or equal to (`<=`): Compares if the first value is less than or equal to the second value.
**Code Block:**
```matlab
a = 5;
b = 3;
% Comparing if a and b are equal
result1 = (a == b); % Result: false
% Comparing if a and b are not equal
result2 = (a ~= b); % Result: true
% Comparing if a is greater than b
result3 = (a > b); % Result: true
```
**Logical Analysis:**
* The first line assigns the variable `a` the value of 5 and the variable `b` the value of 3.
* The second line uses the `==` operator to compare if `a` and `b` are equal, resulting in `false` because `a` does not equal `b`.
* The third line uses the `~=` operator to compare if `a` and `b` are not equal, resulting in `true` because `a` does not equal `b`.
* The fourth line uses the `>` operator to compare if `a` is greater than `b`, resulting in `true` because `a` is greater than `b`.
### 2.2 Logical Operators
Logical operators are used to combine multiple conditions and return a Boolean value. These operators include:
- Logical AND (`&&`): Returns true if all conditions are true.
- Logical OR (`||`): Returns true if any condition is true.
- Logical NOT (`~`): Reverses the Boolean value of the condition.
**Code Block:**
```matlab
% Define conditions
cond1 = a > 0; % a is greater than 0
cond2 = b < 10; % b is less than 10
% Use logical AND operator
result1 = (cond1 && cond2); % Result: true
% Use logical OR operator
result2 = (cond1 || cond2); % Result: true
% Use logical NOT operator
result3 = ~cond1; % Result: false
```
**Logical Analysis:**
* The first line defines two conditions: `cond1` and `cond2`.
* The second line uses the `&&` operator to combine `cond1` and `cond2`. If both conditions are true, the result is true. In this case, both `cond1` and `cond2` are true, so `result1` is true.
* The third line uses the `||` operator to combine `cond1` and `cond2`. If any condition is true, the result is true. In this case, both `cond1` and `cond2` are true, so `result2` is true.
* The fourth line uses the `~` operator to reverse the Boolean value of `cond1`. In this case, `cond1` is true, so `result3` is false.
### 2.3 Nested if Statements
Nested if statements allow you to create multiple conditional blocks within a single if statement. This is very useful fo
0
0
相关推荐
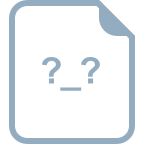
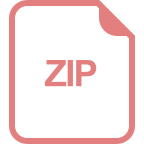
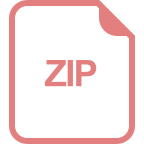
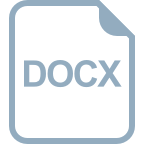
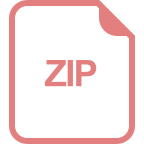
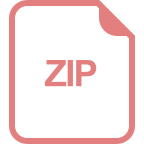
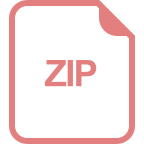