MATLAB Conditional Unit Testing: Ensuring Reliability of Conditional Judgments (with 10 Practical Cases)
发布时间: 2024-09-13 18:24:11 阅读量: 8 订阅数: 18 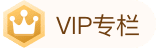
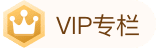
# Introduction to MATLAB Conditional Unit Testing: Ensuring Reliability of Conditional Judgments (with 10 Practical Examples)
## 1. Introduction to MATLAB Conditional Unit Testing
MATLAB conditional unit testing is a method to verify whether conditional judgments in MATLAB code work as intended. It evaluates the correctness of conditional statements by creating test cases, ensuring the code can execute reliably under various inputs and scenarios. Conditional unit testing is crucial for enhancing code quality, reducing the risk of defects, and strengthening the robustness of programs.
## 2. Theoretical Foundations of Conditional Unit Testing
### 2.1 Condition Coverage and Path Coverage
**Condition Coverage**
Condition coverage is a test coverage measure that assesses whether test cases cover all possible branches of all conditional statements in a program. For instance, for an `if` statement, condition coverage requires test cases to cover both the true and false branches of the statement.
**Path Coverage**
Path coverage is a stricter test coverage measure that assesses whether test cases cover all possible execution paths in a program. It requires test cases to cover all possible paths from the start to the end of the program.
### 2.2 Conditional Unit Testing Strategies
**Full Condition Coverage**
Full condition coverage is a conditional unit testing strategy that requires test cases to cover all possible branches of all conditional statements in a program. This is the strictest condition coverage strategy, ensuring that all conditional statements in the program have been tested.
**Partial Condition Coverage**
Partial condition coverage is a conditional unit testing strategy that requires test cases to cover all possible branches of at least one conditional statement in the program. This is a looser strategy than full condition coverage but can still detect many conditional errors.
### 2.3 Test Case Design Techniques
**Boundary Value Analysis**
Boundary value analysis is a test case design technique that generates test cases around the boundary values of conditional statements. For example, for a comparison operator, boundary value analysis would generate test cases to test the equal and not equal branches of the operator.
**Equivalence Class Partitioning**
Equivalence class partitioning is a test case design technique that divides input data into equivalence classes and then generates a test case for each equivalence class. For instance, for a function that accepts integer inputs, equivalence class partitioning would divide the input into positive integers, negative integers, and zero.
**Error Guessing**
Error guessing is a test case design technique that generates test cases based on guesses about where the program might go wrong. For example, for a calculation function, error guessing might generate a test case to test whether the function handles negative inputs.
## 3. Practical Methods for Conditional Unit Testing
### 3.1 MATLAB Unit Testing Framework
MATLAB provides an in-built unit testing framework that allows users to write and run unit tests. The main components of the framework include:
- **Unit test functions:** Functions that define test cases, starting with `test`.
- **Assertion functions:** Functions used to verify test results, such as `assertEqual`, `assertLessThan`, etc.
- **Test suite:** A collection of multiple unit test functions, used for organizing and running tests.
- **Test runner:** Executes the test suite and generates a test report.
### 3.2 Conditional Unit Testing Steps
The steps for conditional unit testing are as follows:
1. **Identify conditional judgments:** Determine which conditional judgments in the
0
0
相关推荐
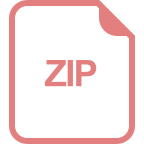
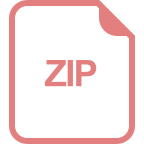
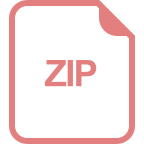





