Conditional Debugging in MATLAB: In-depth Analysis of Conditional Execution (With 15 Real-Life Examples)
发布时间: 2024-09-13 18:23:07 阅读量: 22 订阅数: 23 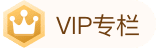
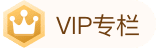
# 1. Introduction to Conditional Debugging in MATLAB**
Conditional debugging is a vital debugging technique in MATLAB, used to identify and resolve errors within conditional statements. Conditional statements govern the flow of program execution, hence debugging them is essential to ensure that programs run as intended.
MATLAB provides a suite of tools and techniques to support conditional debugging, including breakpoints, step-by-step execution, variable inspection, and conditional coverage analysis. By leveraging these tools, debuggers can gain a deep understanding of the execution flow of conditional statements, detect errors, and devise effective solutions.
Conditional debugging is crucial for addressing various common errors such as overly nested conditional statements, improper use of logical operators, and undefined variables. By mastering conditional debugging techniques, MATLAB users can enhance the quality, reliability, and maintainability of their code.
# 2. Theoretical Foundations of Conditional Judgments
### 2.1 Syntax and Semantics of Conditional Statements
Conditional statements are used in MATLAB to control the flow of program execution. The basic syntax is as follows:
```
if condition
statements
end
```
Where:
- `condition` is a logical expression that evaluates to either `true` or `false`.
- `statements` is the block of code to be executed when `condition` is `true`.
Conditional statements also support `elseif` and `else` clauses, allowing for different blocks of code to be executed based on multiple conditions. The syntax is as follows:
```
if condition1
statements1
elseif condition2
statements2
else
statementsN
end
```
### 2.2 Logical Operators and Conditional Expressions
Logical operators are used to combine multiple logical expressions into more complex conditions. The commonly used logical operators in MATLAB include:
- `&&` (AND): The result is `true` only if all operands are `true`.
- `||` (OR): The result is `true` if any operand is `true`.
- `~` (NOT): It negates the value of the operand.
A conditional expression is an expression that combines logical expressions using logical operators. Conditional expressions can serve as the `condition`, or as a component of other expressions.
### 2.3 Execution Flow of Conditional Judgments
The execution flow of a conditional statement is as follows:
1. Evaluate the value of `condition`.
2. If `condition` is `true`, execute the `statements` block.
3. If `condition` is `false`, execute the `elseif` clause or the `else` clause (if present).
4. If there are no matching `elseif` or `else` clauses, skip the conditional statement.
**Code Block:**
```
% Define variables
x = 5;
y = 10;
% Use if-else statement to determine the relationship between x and y
if x > y
disp('x is greater than y')
else
disp('x is not greater than y')
end
```
**Logical Analysis:**
This code block defines two variables, `x` and `y`, and then uses an `if-else` statement to determine if `x` is greater than `y`. If `x` is greater, it outputs "x is greater than y", otherwise, it outputs "x is not greater than y".
**Parameter Explanation:**
- `x`: The first variable to be compared.
- `y`: The second variable to be compared.
# 3.1 Using Breakpoints and Step-by-Step Execution
A breakpoint is a debugging tool that allows you to pause execution when the program reaches a specific line. This enables you to inspect variable values, evaluate expression results, and analyze the execution path of conditional judgments.
**Setting Breakpoints**
In MATLAB, breakpoints can be set in the following ways:
- Place the cursor on the line where you want to set a breakpoint and press F9.
- Click the "Breakpoints" button on the debugging toolbar and select "Set breakpoint on current line".
**Step-by-Step Execution**
Step-by-step execution allows you to execute the code line by line, which aids in tracking changes to variable values and the flow of conditional judgments.
- Click the "Step Execution" button on the debugging toolbar.
- Press F10 to step to the next line.
- Press F11 to step into the next statement.
**Example of Using Breakpoints and Step-by-Step Execution**
The following example demonstrates how to use breakpoints and step-by-step execution to debug conditional judgments:
```matlab
% Define variables
x = 5;
y = 10;
% Set a breakpoint
setdbstops('at', 7);
% Execute code
if x > y
fprintf('x is greater than y\n');
else
fprintf('x is not greater than y\n');
end
```
When the code reaches line 7, MATLAB will pause execution at the breakpoint. You can use the "Variables" window to inspect the v
0
0
相关推荐
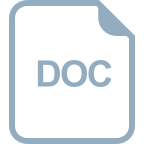
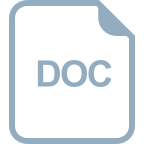
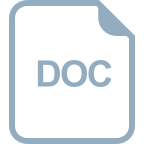
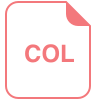
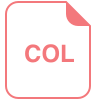
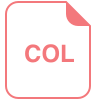
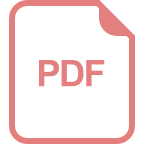
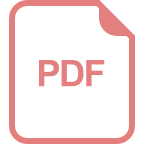
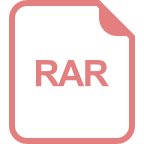