Refactoring Conditional Code in MATLAB: Enhancing Readability and Maintainability of Conditional Logic (with 10 Practical Examples)
发布时间: 2024-09-13 18:27:19 阅读量: 28 订阅数: 27 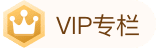
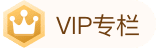
# Refactoring Conditional Code in MATLAB: Enhancing Readability and Maintainability with 10 Practical Examples
## 1. The Basics of Conditional Statements
Conditional statements are fundamental structures that control the flow of a program in MATLAB. They allow the program to execute different blocks of code based on specific conditions. The syntax for a conditional statement is:
```matlab
if condition
statements
end
```
Here, `condition` is a boolean expression, and `statements` are the code blocks executed when the condition is true. If the condition is false, the `statements` are skipped.
Conditional statements can be nested to form complex decision structures. For example:
```matlab
if condition1
statements1
else
if condition2
statements2
else
statements3
end
end
```
Conditional statements enable programmers to make decisions based on input data or the state of the program, thereby controlling the execution flow of the program.
## 2. Principles of Conditional Code Refactoring
### 2.1 Readability Principle
The **Readability Principle** emphasizes clarity and ease of understanding in conditional code. Following these guidelines can improve readability:
- **Use clear variable and function names:** Variable names and function names should accurately reflect their meanings, avoiding abbreviations or ambiguous names.
- **Avoid nested conditions:** Nested conditions can make code difficult to read and understand. Try to break down conditions into smaller blocks.
- **Consistent indentation and formatting:** Consistent indentation and formatting help improve the readability of code, making its structure clear.
- **Add comments:** Add comments when necessary to explain complex conditions or algorithms, enhancing the comprehensibility of the code.
### 2.2 Maintainability Principle
The **Maintainability Principle** focuses on the ease of modifying and extending code. Following these guidelines can improve maintainability:
- **Use modular design:** Organize conditional code into independent modules or functions to increase code reusability and maintainability.
- **Avoid hardcoding:** Avoid embedding conditional values directly in the code. Use parameters or configuration files to store conditional values, making the code easier to modify.
- **Use exception handling:** Handle exceptions that may occur in conditional code to ensure the code runs correctly in unexpected situations.
- **Perform unit testing:** Write unit tests to verify the correctness of conditional code, increasing the reliability of the code.
### 2.3 Performance Principle
The **Performance Principle** focuses on the efficiency of the execution of conditional code. Following these guidelines can improve performance:
- **Avoid unnecessary condition checks:** Only check necessary conditions to avoid unnecessary computation and branching.
- **Use short-circuit evaluation:** Use short-circuit evaluation operators (&& and ||) to improve the efficiency of condition checking.
- **Use caching:** Cache results for frequently calculated conditions to avoid repeated computation.
- **Consider compiler optimizations:** Use compiler optimization options, such as loop unrolling and inlining, to improve the performance of conditional code.
**Code Examples:**
```matlab
% Readability Principle
if (x > 0) && (y < 0)
% Code block 1
elseif (x < 0) && (y > 0)
% Code block 2
else
% Code block 3
end
% Maintainability Principle
function is_valid_input(x, y)
if (x > 0) && (y < 0)
return true;
elseif (x < 0) && (y > 0)
return true;
else
return false;
end
end
% Performance Principle
if (x > 0) && (y < 0)
% Code block 1
else
% Code block 2
end
```
**Code Logic Analysis:**
- **Readability Principle:** Using clear variable names (x, y) and explicit condition statements improves code readability.
- **Maintaina
0
0
相关推荐








