In-depth Exploration of switch-case Statements in MATLAB: The Powerhouse for Multiple Condition Judgments (with Real-World Case Studies)
发布时间: 2024-09-13 18:05:44 阅读量: 36 订阅数: 29 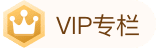
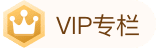
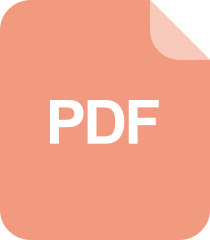
THE RE-EXPLORATION OF THE SPATIAL OSCILLATIONS IN FINITE DIFFERENCE SOLUTIONS FOR NAVIER-STOKES SHOCKS
## In-Depth Exploration of switch-case Statements in MATLAB: A Powerful Tool for Multiple Condition Judgments (with Real-World Examples)
# 1. Overview of switch-case Statements in MATLAB
The switch-case statement in MATLAB is a multi-selection control structure used to execute different code blocks based on given conditions. It is similar to switch statements in other programming languages but comes with MATLAB-specific syntax and functionalities.
The switch-case statement works by comparing an expression with a series of case values. If the expression matches any of the case values, the code block associated with that case is executed. If there is no match, the otherwise code block (if present) is executed.
# 2. Syntax and Usage of switch-case Statements
### 2.1 Syntax Structure of switch Statements
```matlab
switch expression
case value1
statements1
case value2
statements2
...
otherwise
statements_otherwise
end
```
Where:
- `expression`: The expression to be evaluated, which can be any scalar value (number, character, string, or logical value).
- `case`: Specifies the specific value to match.
- `value`: The specific value to match.
- `statements`: The statements to execute when the value of `expression` matches the `value` in `case`.
- `otherwise`: The statements to execute when the value of `expression` does not match any value in `case` (optional).
### 2.2 Syntax Structure of case Statements
```matlab
case value1
statements1
```
Where:
- `value`: The specific value to match.
- `statements`: The statements to execute when the value of `expression` matches the `value` in `case`.
### 2.3 Role of break Statements
The `break` statement is used to exit the `switch` statement and continue executing the code following the `switch` statement. If the `break` statement is not used, the `switch` statement will continue executing the statements in the next `case` statement, even if the value of `expression` does not match the `value` in that `case`.
### 2.4 Role of otherwise Statements
The `otherwise` statement is used to specify the statements to execute when the value of `expression` does not match any value in `case`. If the `otherwise` statement does not exist, the `switch` statement will not perform any actions when the value of `expression` does not match any `case`.
**Example:**
```matlab
switch input('Please enter a number: ')
case 1
disp('You entered the number 1')
case 2
disp('You entered the number 2')
case 3
disp('You entered the number 3')
otherwise
disp('You entered an invalid number')
end
```
**Logical Analysis:**
This code creates a simple menu where users can enter a number. It then uses a `switch` statement to perform different actions based on the user's input value. If the user enters the numbers 1, 2, or 3, corresponding messages will be displayed. If the user enters any other value, an error message will be shown.
# 3.1 Simple Multiple Condition Judgment
One of the most common applications of switch-case statements is to make multiple condition judgments. In MATLAB, you can use switch-case statements to compare a variable and execute different code blocks based on different matching results.
For example, suppose we have a variable `choice` that can be 1, 2, or 3. We can use a switch-case statement to determine the value of `choice` and execute different operations based on the value:
```matlab
choice = 1;
switch choice
case 1
disp('You chose option 1');
case 2
disp('You chose option 2');
case 3
disp('You chose option 3');
otherwise
disp('You entered an invalid option');
end
```
After running this code, the following content will be output:
```
You chose option 1
```
### 3.2 Executing Different Operations Based on Input Value
Switch-case statements can also be used to execute different operations based on input values. For example, we can use switch-case statements to handle user input commands:
```matlab
command = input('Please enter a command (1: view help, 2: exit): ', 's');
switch command
case '1'
disp('Help information');
case '2'
disp('Exit program');
otherwise
disp('Invalid command');
end
```
After running this code, users can input a command (1 or 2), and the program will execute different operations based on the user's input.
### 3.3 Nested switch-case Statements
Switch-case statements can be nested to handle more complex conditional judgments. For example, suppose we have a variable `choice1`, which can be 1 or 2, and another variable `choice2`, which can be 1, 2, or 3. We can use nested switch-case statements to determine the values of `choice1` and `choice2` and execute different operations based on the different combinations:
```matlab
choice1 = 1;
choice2 = 2;
switch choice1
case 1
switch choice2
case 1
disp('You chose option 1-1');
case 2
disp('You chose option 1-2');
case 3
disp('You chose option 1-3');
otherwise
disp('You entered an invalid option');
end
case 2
switch choice2
case 1
disp('You chose option 2-1');
case 2
disp('You chose option 2-2');
case 3
disp('You chose option 2-3');
otherwise
disp('You entered an invalid option');
end
otherwise
disp('You entered an invalid option');
end
```
After running this code, the following content will be output:
```
You chose option 1-2
```
# 4. Advanced Techniques for switch-case Statements
### 4.1 Using Regular Expressions to Match Conditions
In some cases, you may need to use regular expressions to match conditions. Regular expressions are a powerful pattern-matching language that can be used to find and extract patterns in strings.
```matlab
switch regexprep(input('Enter a string: '), '[^a-zA-Z]', '')
case 'hello'
disp('Hello, world!')
case 'goodbye'
disp('Goodbye, world!')
otherwise
disp('Invalid input')
end
```
**Code Logic Analysis:**
* The `regexprep` function is used to replace all non-letter characters in the input string with an empty string, thus creating a string that only contains letters.
* The `switch` statement uses regular expressions to match conditions.
* If the input string matches "hello" or "goodbye," the corresponding `case` statements are executed.
* If the input string does not match any `case` statements, the `otherwise` statement is executed.
### 4.2 Using cell Arrays as Conditions
You can also use cell arrays as the conditions for `switch` statements. A cell array is an array that can store different types of data.
```matlab
conditions = {'hello', 'goodbye', 'thank you'};
switch input('Enter a word: ')
case conditions
disp('Valid input')
otherwise
disp('Invalid input')
end
```
**Code Logic Analysis:**
* The `conditions` variable is a cell array containing three strings.
* The `switch` statement uses a cell array as a condition.
* If the input word matches any element in the `conditions` array, the `case` statement is executed.
* If the input word does not match any element in the `conditions` array, the `otherwise` statement is executed.
### 4.3 Using Anonymous Functions as Conditions
Anonymous functions are functions without a name and can be used as conditions for `switch` statements.
```matlab
switch input('Enter a number: ')
case @(x) x > 0
disp('Positive number')
case @(x) x < 0
disp('Negative number')
otherwise
disp('Zero')
end
```
**Code Logic Analysis:**
* The `switch` statement uses an anonymous function as a condition.
* The first `case` statement checks if the input number is greater than 0.
* The second `case` statement checks if the input number is less than 0.
* If the input number does not satisfy any `case` statements, the `otherwise` statement is executed.
# 5. Performance Optimization of switch-case Statements
When using switch-case statements, performance optimization is an important consideration. Here are some tips for optimizing the performance of switch-case statements:
### 5.1 Avoid Using Too Many case Statements
Too many case statements can degrade the performance of switch-case statements. This is because MATLAB needs to compare the input value with the condition of each case statement one by one when executing the switch statement. The more case statements there are, the more comparisons are needed, and the worse the performance will be.
To avoid using too many case statements, consider the following techniques:
- **Merge similar case statements:** If there are multiple case statements with very similar conditions, you can merge them into one case statement. For example, the following two case statements can be merged into one:
```matlab
switch x
case 1
% Perform operation 1
case 2
% Perform operation 2
end
```
```matlab
switch x
case {1, 2}
% Perform operation 1 or operation 2
end
```
- **Use range conditions:** Range conditions allow you to specify a range of values instead of a single value. This can reduce the number of case statements. For example, the following three case statements can be merged into one:
```matlab
switch x
case 1
% Perform operation 1
case 2
% Perform operation 2
case 3
% Perform operation 3
end
```
```matlab
switch x
case 1:3
% Perform operation 1, 2, or 3
end
```
### 5.2 Use the fallthrough Keyword
The fallthrough keyword allows you to continue executing the next case statement after executing one case statement. This can avoid using the `break` statement in each case statement, thus improving performance.
For example, the following two case statements use the fallthrough keyword to merge into one:
```matlab
switch x
case 1
% Perform operation 1
fallthrough;
case 2
% Perform operation 2
end
```
### 5.3 Use the switch Function
In some cases, using the switch function is more efficient than using the switch-case statement. The switch function is a built-in function that accepts an input value and a cell array containing case statements. The switch function compares the input value with each case statement's condition one by one and performs the operation associated with the first matching case statement.
For example, the following switch-case statements can be converted to a switch function:
```matlab
switch x
case 1
% Perform operation 1
case 2
% Perform operation 2
case 3
% Perform operation 3
end
```
```matlab
cases = {1, 2, 3};
actions = {@() disp('Perform operation 1'), @() disp('Perform operation 2'), @() disp('Perform operation 3')};
switchFunction(x, cases, actions);
```
The advantage of the switch function is that it avoids comparing the input value with each case statement's condition one by one. This can improve performance, especially when there are a large number of case statements.
# ***mon Problems and Solutions for switch-case Statements
### 6.1 No match for any case statements
If none of the case statements in the switch statement match the input value, the otherwise statement will be executed. If the otherwise statement is not provided, an error will be thrown.
**Solution:**
* Add an otherwise statement to handle unmatched input values.
* Carefully check the conditions of the case statements to ensure they cover all possible scenarios.
### 6.2 No break statement in case statements
If there is no break statement in a case statement, the subsequent case statements will be executed until a break statement or otherwise statement is encountered. This can lead to unexpected results.
**Solution:**
* Add a break statement at the end of each case statement to prevent the execution of subsequent case statements.
### 6.3 Best Practices for Using switch-case Statements
***Use switch-case statements for multiple condition judgments:** When you need to perform different actions based on multiple conditions, you can use `switch-case` statements.
***Keep case statements concise:** Each `case` statement should only contain one condition. If a condition is complex, you can split it into multiple `case` statements.
***Use the fallthrough keyword:** If you need to execute multiple consecutive case statements, you can use the `fallthrough` keyword.
***Use the switch function:** For simple multiple condition judgments, you can use the `switch` function, which returns the value corresponding to the matching case statement.
***Avoid overusing switch-case statements:** If a `switch-case` statement becomes too complex, consider using other control flow structures, such as `if-else` statements or `for` loops.
0
0
相关推荐







