Truth Table Analysis: From Simple to Complex, In-depth Exploration of Logical Operations (10 Practical Cases)
发布时间: 2024-09-15 09:08:15 阅读量: 28 订阅数: 20 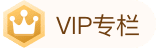
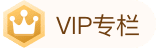
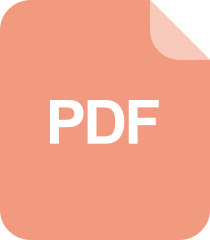
From Device To System: Cross-Layer Design Exploration of Racetrack Memory
# Concept and Basic Operations of Truth Tables
A truth table is a table that displays the output values of logical operators for all possible combinations of inputs. In a truth table, input values are typically represented by Boolean variables, which can only take two values: true (True) or false (False).
The basic logical operators include:
- AND operation (AND): The output is true only when all inputs are true.
- OR operation (OR): The output is true if at least one input is true.
- NOT operation (NOT): The output is false when the input is true; the output is true when the input is false.
# 2.1 Boolean Algebra Theorems and Their Applications
### 2.1.1 Identity Law, Contradiction Law, and Excluded Middle Law
In Boolean algebra, the identity law, contradiction law, and excluded middle law are three basic theorems that define the fundamental properties of logical operations.
**Identity Law:**
```
A ∨ A = A
A ∧ A = A
```
**Explanation:** The result of any logical variable undergoing a logical OR or logical AND operation with itself is always equal to itself.
**Contradiction Law:**
```
A ∨ ¬A = 1
A ∧ ¬A = 0
```
**Explanation:** The result of any logical variable undergoing a logical OR operation with its negation is always true; the result of undergoing a logical AND operation with its negation is always false.
**Excluded Middle Law:**
```
¬(¬A) = A
```
**Explanation:** The result of any logical variable undergoing double negation is always equal to itself.
### 2.1.2 Distributive Law, Associative Law, and Commutative Law
The distributive law, associative law, and commutative law are three other important theorems in Boolean algebra that define the relationships between logical operations.
**Distributive Law:**
```
A ∨ (B ∧ C) = (A ∨ B) ∧ (A ∨ C)
A ∧ (B ∨ C) = (A ∧ B) ∨ (A ∧ C)
```
**Explanation:** The logical OR operation is distributive over the logical AND operation, and the logical AND operation is distributive over the logical OR operation.
**Associative Law:**
```
(A ∨ B) ∨ C = A ∨ (B ∨ C)
(A ∧ B) ∧ C = A ∧ (B ∧ C)
```
**Explanation:** Both the logical OR and logical AND operations are associative, meaning multiple operations can be grouped arbitrarily.
**Commutative Law:**
```
A ∨ B = B ∨ A
A ∧ B = B ∧ A
```
**Explanation:** Both the logical OR and logical AND operations are commutative, meaning the order of operations can be swapped.
### Applications
Boolean algebra theorems have a wide range of applications in logical operations, including:
***Circuit Design:** Boolean algebra theorems are used to design and simplify logical circuits.
***Computer Programming:** Boolean algebra theorems are used to write logical judgments and control statements.
***Mathematical Proofs:** Boolean algebra theorems are used to prove logical propositions and theorems.
***Artificial Intelligence:** Boolean algebra theorems are used to represent and reason about knowledge.
# 3.1 Truth Tables in Digital Circuit Design
**3.1.1 Truth Tables for Combinational Logic Circuits**
A combinational logic circuit is a type of logic circuit where the output is solely dependent on its current inputs. Its truth table describes the circuit's output values for all possible combinations of inputs. For example, a simple AND gate circuit with two inputs A and B has the following truth table:
| A | B | Output |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
From the truth table, we can see that the AND gate only outputs a 1 when both A and B are 1.
**3.1.2 Truth Tables for Sequential Logic Circuits**
The output of a sequential logic circuit depends not only on its current inputs but also on its past states. Its truth table describes the circuit's output values for all possible combinations of inputs and states. For example, a simple D flip-flop circuit with an input D and a clock input CLK has the following truth table:
| Current State | CLK | D | Next State | Output |
|---|---|---|---|---|
| 0 | 0 | X | 0 | 0 |
| 0 | 1 | 0 | 0 | 0 |
| 0 | 1 | 1 | 1 | 1 |
| 1 | 0 | X
0
0
相关推荐
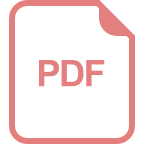
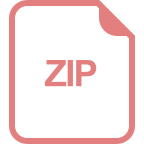
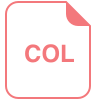
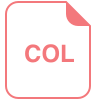
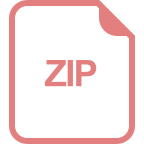
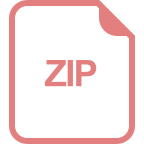
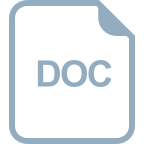
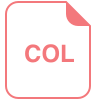
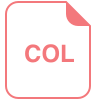